Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial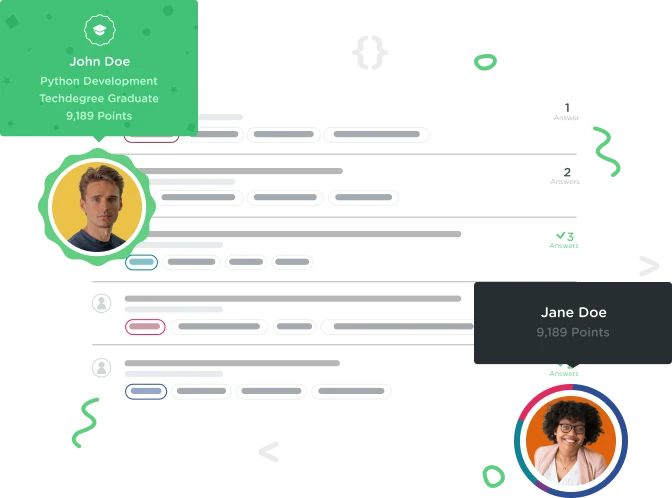

Jonathan Nott
538 PointsWhat is happening here? why is their a letter m before the variable? public String mColor
Here is the Class:
public class GoKart { public String mColor = "red";
public String getColor(){ return mColor; } }
2 Answers
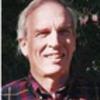
jcorum
71,830 PointsMany consider it "best practice" to prefix instance variables with the letter m. It's not required, but you will see it a lot in these videos and challenges.
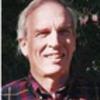
jcorum
71,830 PointsSorry, but there really are no standard variables. Variables can either be class (static) variables, or instance variables.
public class Circle {
private double radius; //instance variable
private static int numCircles = 0; //class variable
public Circle(double radius) { //constructor
this.radius = radius;
numCircles++; //keep track of how many circles are created
}
public int getNumCircles() { //getter method for numCircles
return numCircles;
}
public static void main(String[] args) {
Circle c1 = new Circle(23.5);
System.out.println(c1.getNumCircles()); // prints 1
}
}
In the above code, whenever a new circle object is created (as an instance of Circle), it has its own copy of the instance variables (here radius, which is the only one). You can see why: every circle can and may have a different radius, which is specified when you call the constructor.
Class variables are different. There is only one value, which is shared by every object created from the class. Again, you can see why: it wouldn't be very valuable information if every circle had a different value for numCircles. Every Circle object can read the class variable numCircles, because of the getter method, but there is only one value at any given time. If there are 10 Circle objects then numCircles would be 10. If there are 100, numCircles would be 100.
Note that it would be a big mistake to write a setter method for numCircles.
Jonathan Nott
538 PointsJonathan Nott
538 PointsGreat, thanks.
That opens a new question, how do you best describe an instance variable and how is it different to a standard variable?