Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial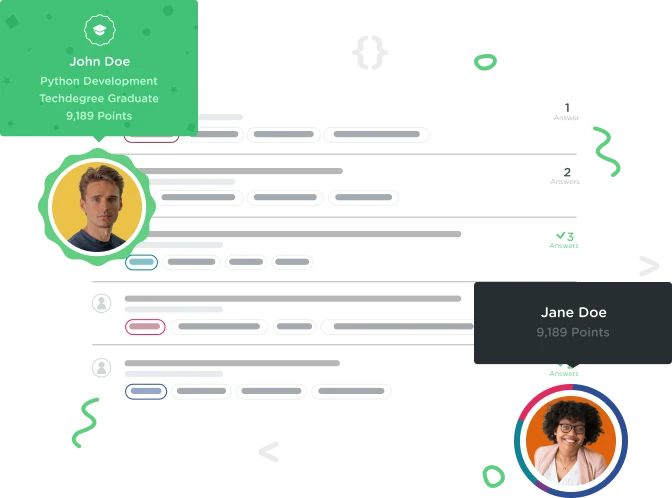

Christopher Clemmons
3,476 PointsWhat is _mayor referring to?
I am having an issue understanding what _mayor is referring to. The parameter or the actual setter?
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major){
if(this.level === "Senior" || this.level === "Junior"){
this._major = major;
} else { return this._mayor = 'None'; }
}
}
var student = new Student(3.9, 60);
1 Answer

Simon Coates
8,177 PointsI think, using the 'this' keyword means you're operating on the copy stored on the object. It's not the parameter and it's not the setter, it's a backing field. But if should be '_major' not '_mayor'. Here's another example of a getter and setter.
class User {
constructor(name) {
// invokes the setter
this.name = name;
}
get name() {
return this._name;
}
set name(value) {
if (value.length < 4) {
alert("Name is too short.");
return;
}
this._name = value;
}
}
let user = new User("John");
alert(user.name); // John
user._name; //outputs "John"
In this instance, you're meant to interact with the getter and setter, but it demos that there's a field behind the getters and setters.