Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial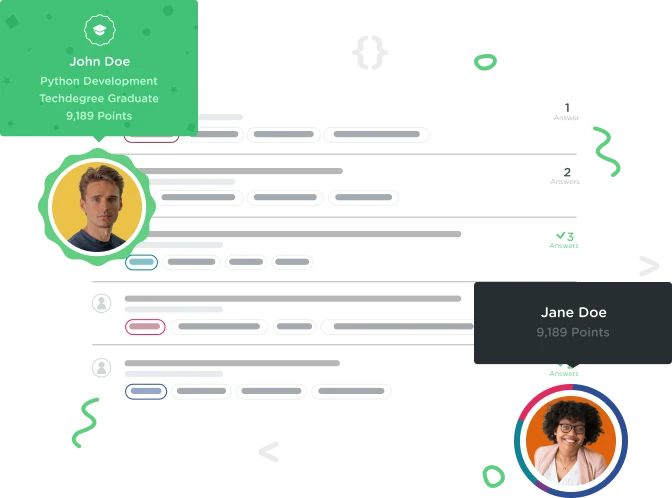
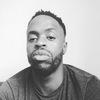
olu adesina
23,007 Pointswhat is meant by the index was out of the bounds of the array
i think that i have completed the objective but it keeps telling me the index was out of the bounds of the array
not sure where i'm going wrong here any help
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector :SequenceDetector
{
public int y = 1;
public override bool Scan(int[] sequence)
{
for (int i = 0; i < sequence.Length; i++)
{
if (sequence[i] == sequence[y])
{
return true;
}
else
{
y++;
}
}
return false;
}
}
}
3 Answers
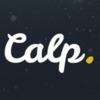
calp
10,317 PointsIf it's telling you that the index is out of bounds when you're trying to access a value that is too large or too small for your array. For example, if you have an array that has a capacity of 3 items, you may be trying to access the fourth item (which is out of bounds and does not exist).
It's more than likely your loop is trying to access a part of the array that doesn't exist.
I've had a quick look at your code and the Y variable starts at 1 and is then continuously incremented inside of the loop. This will result in that variable is one larger than the array. I suggest adjusting that or adjusting your loop to stop at i < sequence.Length - 1
Goodluck
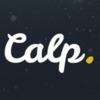
calp
10,317 PointsI just modified your code a bit and was able to pass the challenge.
namespace Treehouse.CodeChallenges
{
class RepeatDetector :SequenceDetector
{
public override bool Scan(int[] sequence)
{
for (int i = 0; i < sequence.Length; i++)
{
if (sequence[i] == sequence[i+1])
{
return true;
}
}
return false;
}
}
}

Mark VonGyer
21,239 PointsThat code will still throw an exception if the expression never equates to true.
You can probably do with putting the sequence.Length - 1 as you suggested earlier.
You wont need to test the last element in the array because it needs an element after to compare with.
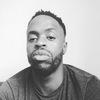
olu adesina
23,007 Pointsi ran it through the vs debugger and managed to sorted it out
namespace ClassLibrary1
{
public class RepeatDetector
{
public int y = 1;
public bool Scan(int[] sequence)
{
for (int i = 0; i < sequence.Length; i++)
{
if (sequence[i] == sequence[y] && i != y)
{
return true;
}
if (y < sequence.Length-1)
{
y++;
}
}
return false;
}
}
}
Mark VonGyer
21,239 PointsMark VonGyer
21,239 PointsThat will fix his problem but I don't think it will be the end of his issues, as it would mean the final element in the array is not tested.
It seems like the function is trying to test for duplicate elements?
Would it not be better to iterate through the elements in the array and check if the first index of it's occurrence matches the last index of its occurrence?
if(sequence.lastIndexOf(sequence[i]) == sequence.indexOf(sequence[i]) return true;
I'm not sure if this is what you are trying to achieve however.