Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial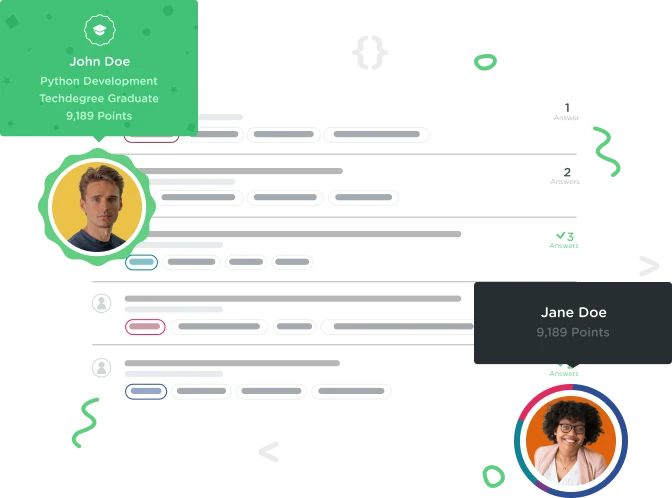

aimensasi
11,343 Pointswhat is Redirect URI?
I am building an application that uses Spotify SDK. I ran into something called redirect URI and it is necessary when registering the app in the Spotify developer https://developer.spotify.com/my-applications. The question is what is Redirect URI? and How to Create one?
Thanks in advance.
3 Answers
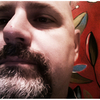
Jeremy Faith
Courses Plus Student 56,696 PointsThe redirect uri is where the client will get send to after the account authorization is successful. You could also set up a redirect for an authorization failure. I have not used the Spotify API but looking at the documents it appears that when you register your application you will add a redirect uri to the white-list. Then in your application when you make a GET request following the format from Spotify's Web API you will include the client id and the redirect uri you want to use for the callback, enrty point back into your application. It must be on the white-list from when you registered your application with Spotify.
I hope this very general overview helped you a little.
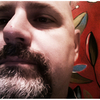
Jeremy Faith
Courses Plus Student 56,696 PointsIt is hard to know what exactly went wrong without seeing all of your code. When you set up your app did you name your Redirect URI in this format yourcustomprotocol://callback. Also, in your MainActivity did you add private static final String REDIRECT_URI = "yourcustomprotocol://callback";.
Right now yourcustomprotocol is SpotifyTestApp.
Your callback should redirect back to your app.
It is something that you have to create as part of your APP, for example your callback may be authenticationResponse.
You would then have REDIRECT_URI = "SpotifyTestApp://authenticationResponse"
Again, I have not used the Spotify WEB API before and I am just going through the documentation. Still, I hope this helps you move in the right direction.

aimensasi
11,343 PointsThanks again for your time. This my full code :
MainAvtivity:
package com.spotifycallback.spotify;
import android.app.Activity; import android.content.Intent; import android.net.Uri; import android.os.Bundle; import android.util.Log;
import com.spotify.sdk.android.player.Spotify; import com.spotify.sdk.android.authentication.AuthenticationClient; import com.spotify.sdk.android.authentication.AuthenticationRequest; import com.spotify.sdk.android.authentication.AuthenticationResponse; import com.spotify.sdk.android.player.Config; import com.spotify.sdk.android.player.ConnectionStateCallback; import com.spotify.sdk.android.player.Player; import com.spotify.sdk.android.player.PlayerNotificationCallback; import com.spotify.sdk.android.player.PlayerState;
public class MainActivity extends Activity implements PlayerNotificationCallback, ConnectionStateCallback {
private static final String CLIENT_ID = "a00f335fbd1849bc8841cf3ab3f31b00";
private static final String TAG = MainActivity.class.getSimpleName();
private static final String REDIRECT_URI = "SpotifyTestApp://callback";
private static final int REQUEST_CODE = 1337;
private Player mPlayer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
AuthenticationRequest.Builder builder = new AuthenticationRequest.Builder(CLIENT_ID,
AuthenticationResponse.Type.TOKEN,
REDIRECT_URI);
builder.setScopes(new String[]{"user-read-private", "streaming"});
AuthenticationRequest request = builder.build();
Log.e("Spotify aa " , "the request is sent");
AuthenticationClient.openLoginInBrowser(this, request);
}
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
Uri data = intent.getData();
Log.e(TAG, "data");
if (data != null) {
AuthenticationResponse response = AuthenticationResponse.fromUri(data);
switch (response.getType()) {
// Response was successful and contains auth token
case TOKEN:
// Handle successful response
Log.e(TAG, "the response is success");
break;
// Auth flow returned an error
case ERROR:
Log.e(TAG, "the response is fail");
// Handle error response
break;
// Most likely auth flow was cancelled
default:
// Handle other cases
}
}
}
@Override
protected void onDestroy() {
Spotify.destroyPlayer(this);
super.onDestroy();
}
@Override
public void onLoggedIn() {
Log.d("MainActivity", "User logged in");
}
@Override
public void onLoggedOut() {
Log.d("MainActivity", "User logged out");
}
@Override
public void onLoginFailed(Throwable error) {
Log.d("MainActivity", "Login failed");
}
@Override
public void onTemporaryError() {
Log.d("MainActivity", "Temporary error occurred");
}
@Override
public void onConnectionMessage(String message) {
Log.d("MainActivity", "Received connection message: " + message);
}
@Override
public void onPlaybackEvent(EventType eventType, PlayerState playerState) {
Log.d("MainActivity", "Playback event received: " + eventType.name());
switch (eventType) {
// Handle event type as necessary
default:
break;
}
}
@Override
public void onPlaybackError(ErrorType errorType, String errorDetails) {
Log.d("MainActivity", "Playback error received: " + errorType.name());
switch (errorType) {
// Handle error type as necessary
default:
break;
}
}
}
This The manifest
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.spotifycallback.spotify" >
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<intent-filter>
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.BROWSABLE"/>
<category android:name="android.intent.category.DEFAULT"/>
<data android:scheme="SpotifyTestApp" android:host="callback"/>
</intent-filter>
</activity>
</application>
</manifest>
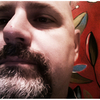
Jeremy Faith
Courses Plus Student 56,696 PointsWell, besides what I already suggested, I can not figure out what is wrong. When you get it working please let me know what the issue is. I am curious what is causing the error.

aimensasi
11,343 PointsThanks for your help.
I tried using >> http://localhost:8888/callback << is a redirect URI and it did work but nothing else work it keep saying either The redirect URI is not valid or No Activity found to handle the callback.
One question is there any side effect of using >> http://localhost:8888/callback << as a redirect URI?
and Thanks again.
aimensasi
11,343 Pointsaimensasi
11,343 PointsThanks so much for your help. One question, how can I create redirect Uri for my application?
aimensasi
11,343 Pointsaimensasi
11,343 PointsI were able to create a Redirect URI following Spotify instruction https://developer.spotify.com/technologies/spotify-android-sdk/android-sdk-authentication-guide/
This my Filter intent:
<intent-filter> <action android:name="android.intent.action.VIEW"/> <category android:name="android.intent.category.BROWSABLE"/> <category android:name="android.intent.category.DEFAULT"/> <data android:scheme="SpotifyTestApp" android:host="callback"/> </intent-filter>
After I finish the log in process the, I ran into an error that says : UNKNOWN_URL_SCHEME
what is the syntax I have to follow explain to me please with example?