Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial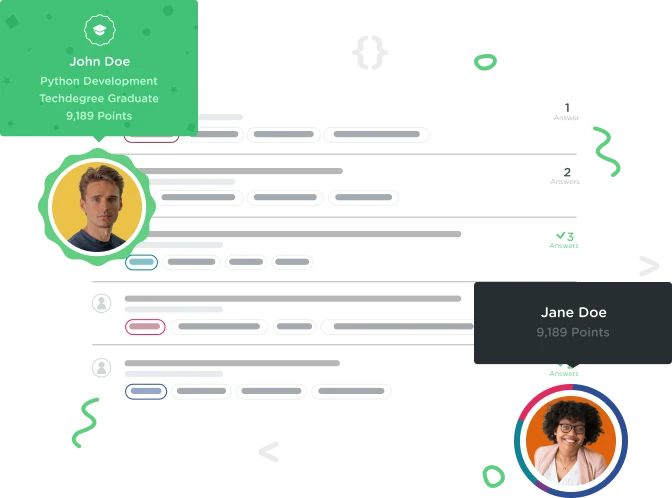
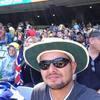
Irfan Hussain
Courses Plus Student 6,593 PointsWhat is State in JavaScript? Specially when working with objects.
What is State in JavaScript? Specially when working with objects.
4 Answers

Thomas Nilsen
14,957 PointsHere is a small example for maintaining 'state' in javascript - through something called closures.
function count() {
//Starting value
var count = 1;
return function() {
//Since 'count' is not defined here
//It goes up the scope-chain searching for it
console.log(count++);
}
}
var count = count();
count(); //outputs 1
count(); // 2
count(); // 3
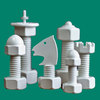
Steven Parker
231,268 PointsHow does this example represent "State"?

Thomas Nilsen
14,957 PointsWhat I did in my example was:
Setting a closure scope and return a function that has access to that scope. Every time that function is called, the state will remain as long as that function exists.
Here is a slightly different example with objects, men the idea is still the same. (This one is from StackOverflow):
var myStatefulObj = function() {
// set up closure scope
var state = 1;
// return object with methods to manipulate closure scope
return {
incr: function() {
state ++;
},
decr: function() {
state--;
},
get: function() {
return state;
}
};
}();
myStatefulObj.incr();
var currState = myStatefulObj.get(); // currState === 2
myStatefulObj.decr();
currState = myStatefulObj.get(); // currState === 1
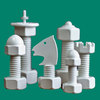
Steven Parker
231,268 PointsSo you're naming the value you get from the internal variable "count" as "currState". But the name is arbitrary. If the closure contained 3 variables instead of just 1, what would you call the other 2?
This is, however, a good example of "scope".

Thomas Nilsen
14,957 Pointsyeah - I agree the naming in my last example was bad (updated it now).
I'm not sure I understand what you mean "...If the closure contained 3 variables instead of just 1, what would you call the other 2"?
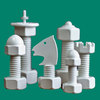
Steven Parker
231,268 PointsI can be more specific when you give an example, but just in general "State" has no special meaning in JavaScript.
You probably saw it used with some code that is written to use it as a variable or passed argument to represent something.
Steven Parker
231,268 PointsSteven Parker
231,268 PointsDo you have an example? The challenge you linked to doesn't seem to use it.