Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial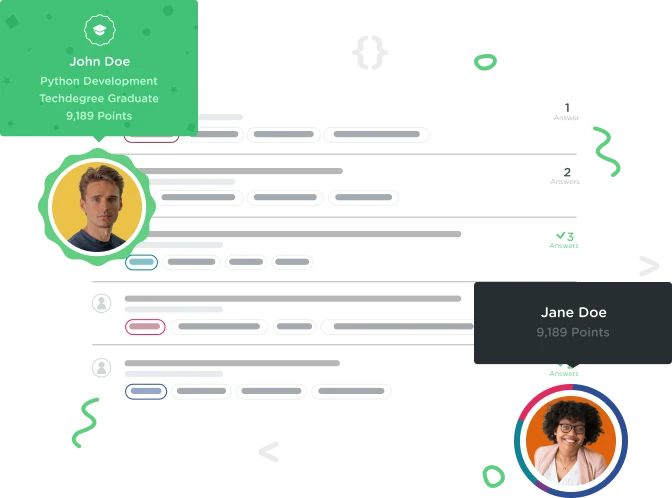
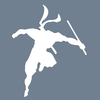
Adrian Melendez
5,555 PointsWhat is the best way to check if a parameter is an array?
The challenge is:
"Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in."
I came up with this code which passed the challenge, but I'm not sure if it is the best way to check if a parameter is an array:
function arrayCounter(ArrayParam) {
if ((typeof ArrayParam === 'string') ||
(typeof ArrayParam === 'number') ||
(typeof ArrayParam === 'undefined')){
return 0;
} else {
return ArrayParam.length;
}
};
The code trys to return the length of any input that isn't a string, number, or undefined. For example, if a function or non-array object were used as the parameter, the arrayCounter function would try to return the length even though the parameter isn't an array.
1 Answer
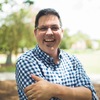
Dave McFarland
Treehouse TeacherYou're right that the code for the challenge won't correctly test for an Array. You're better off using JavaScript's instanceof
operator. It can tell you if a variable is an array or not like this:
function arrayCounter (array) {
if (array instanceof Array) {
return array.length;
} else {
return 0;
}
}
var myArray = [1,2,3];
console.log(arrayCounter(myArray)); // prints 3 to the console
var myString = 'test';
console.log(arrayCounter(myString)); // prints 0 to the console
var myObj = { name: 'object' };
console.log(arrayCounter(myObj)); // prints 0 to the console