Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial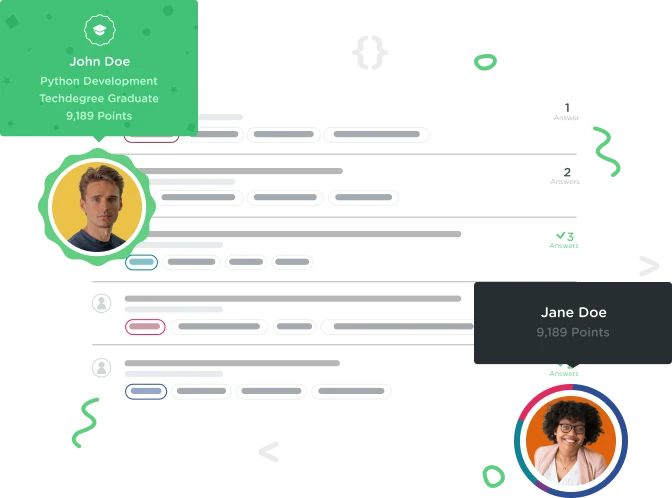
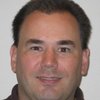
Michael McDonald
2,877 PointsWhat is the correct way to initialize the string array?
I would initialize the array by: titles[1] = title1; titles[2] = title2; titles[3] = title3;
I do not get any compiler errors, just "Bummer! Try again."
I declare the string array with String titles[] = new String[3]; or String[] titles = new String[3];
the output is the same.
String title1 = "Android is awesome";
String title2 = "Mike is cool";
String title3 = "Treehouse loves me";
String[] titles = new String[3];
titles[1] = "Android is awesome";
titles[2] = "Mike is cool";
titles[3] = "Treehouse loves me";
2 Answers

Stone Preston
42,016 Pointsremember that array indexes start at 0, not 1. so when adding the first title to the array you need to use index 1, then for the second use 2 and so on:
String title1 = "Android is awesome";
String title2 = "Mike is cool";
String title3 = "Treehouse loves me";
String[] titles = new String[3];
titles[0] = "Android is awesome";
titles[1] = "Mike is cool";
titles[2] = "Treehouse loves me";
before you started with index 1.
// this starts at index 1, which is the second position in the array. index 0 is the first
titles[1] = "Android is awesome";
titles[2] = "Mike is cool";
titles[3] = "Treehouse loves me";
a somewhat quicker way would be to add the items to the array using {} style syntax where you put the items you want to include in the array between a set of curly braces:
String title1 = "Android is awesome";
String title2 = "Mike is cool";
String title3 = "Treehouse loves me";
String[] titles = { title1, title2, title3 };
you can read more about Arrays and array indexing in the Array documentation
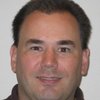
Michael McDonald
2,877 PointsThese are symptoms of the challenge engine detection working too well in previous exercises. :P Thanks again.

Stone Preston
42,016 Pointsno problem
Michael McDonald
2,877 PointsMichael McDonald
2,877 PointsYeah. I do remember that the first in the index is zero, but I expected a compiler error which I didn't get. Your last line is what I really wanted... Thanks.
String[] titles = { title1, title2, title3 };
Stone Preston
42,016 PointsStone Preston
42,016 Pointsyour code would compile fine. there was nothing syntactically wrong with your code, its just your array didnt have anything at index 0. it had stuff at index 1,2, and 3, while the challenge is expecting it to have stuff at 0, 1 and 2.
your original code was perfectly fine Java and there were no "errors", it just wasnt what the challenge was looking for.
a more informative bummer message would have been nice, but there is only so much the challenge engine can detect