Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial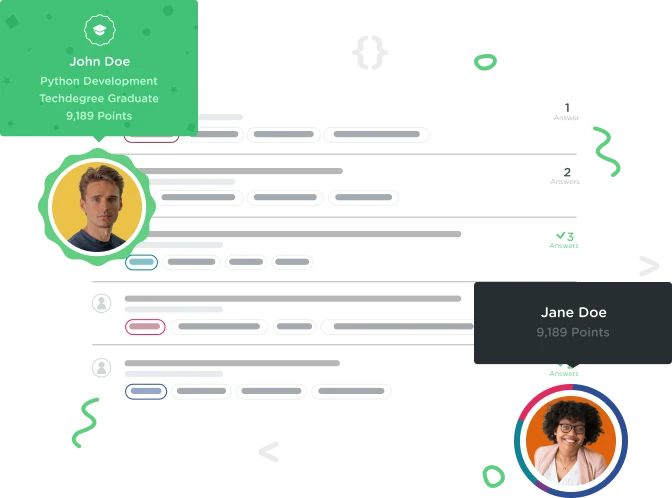

giuseppe prinno
5,060 PointsWhat is the difference between a constructor and a method in java? When do I need one or the other?
When creating an object in java when do I need a constructor and when do I need a method? and what is the difference?
2 Answers
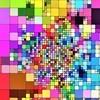
james south
Front End Web Development Techdegree Graduate 33,271 Pointsthe constructor creates the object, methods act on the object. so if for example you had a bank account class, the constructor would create a new bank account. possible methods might be to get the balance, pay the interest, deduct withdrawal amounts etc.

Matthias Margot
7,236 PointsTLDR:
A constructor is used to create an instance of a class. Class methods are callable on created instances of that class.
LONG VERSION:
A constructor does look quite similar to a method and i remember asking myself that very same question for a while when i started getting into this.
The main difference of the two is their name and purpose.
The constructor always has the same name as the class it is a constructor of, so for example if you had a class called Car the constructor could look like this:
access level modifier (public , for example) | class name ( Car , for example ) | ( parameters (String name , for example) ) { something that's done when the constructor is called ( name = mName; , for example) }
public Car (String name) {
name = mName;
}
Here's the thing about object oriented programming: Anything you wanna do in your code is basically executed by calling methods on objects and you can only create those objects by calling the constructor of the class you wanna instantiate an object of.
Like this for example:
Scanner yourScanner = new Scanner(System.in); //variable declaration + calling a constructor of java.util.Scanner
yourScanner.nextLine(); //calling a method on your Scanner object named yourScanner
'yourScanner' is just a variable name you're giving this new Scanner that you're creating with the constructor of the java.util.Scanner class. The '(System.in)' part is a parameter you're giving the constructor so you can create the specific type of Scanner that you need.
After you've done that all you can use all the methods in the java.util.Scanner class to do whatever it is you need to do in your code.
Hope I could help to clarify
Happy coding
Matthias :)

Sarabeth Zogg
9,418 PointsMatthias' answer might be the best explanation I've seen online so far. In the Hangman project, Prompter and Scanner both have constructors but Hangman does not. Is that because nothing is calling on Hangman whereas Hangman calls Prompter and Scanner?

Matthias Margot
7,236 PointsSarabeth Zogg To be honest quite some time has passed since i took that course involving hangman, but i'm guessing that the Hangman class was the one containing the main() method. The code in the main method always runs at least once at the very start of running a java program because that's the starting point of your entire program. It is what the runner (JRE for example) looks for in a program to identify a starting point. So im guessing The hangman class is more of a 'start parameter' class with intructions what the program as a whole has to do and in what order things are executed. No need to ever call a constructor to call the main method that's just done automatically by the runtime environment.
There's one little exception by the way which i didn't mention cause it's not really relevant to the whole method vs. constructor explanation, but there are so called static methods maked with the 'static' keyword which can be called on the class itself with no need of an instantiated object of said class. This is helpful if you wanna make a snippet of code performing some action which doesn't have to be performed on any object, like say some type of unit converter. You just give it something in the parameter and it returns whatever your need. Check out the documentation for java.util.Math, which is a class that doesn't contain anything BUT static methods.