Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial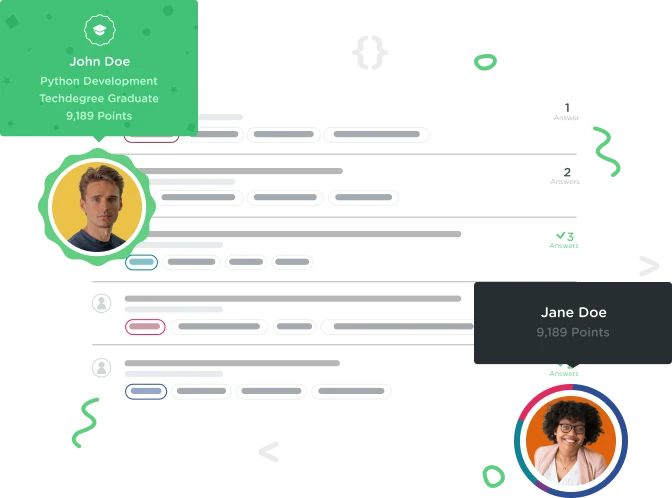

Alex Bargar
760 PointsWhat is the difference between concatenation and interpolation? It seems like you can do the exact same thing with both
You can combine a string and a variable with both. What is the difference then?
2 Answers
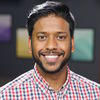
Pasan Premaratne
Treehouse TeacherConcatenation allows you to combine to strings together and it only works on two strings. Swift uses string interpolation to include the name of a constant or variable as a placeholder in a longer string, and to prompt Swift to replace it with the current value of that constant or variable. Some code:
//Concatenation works with strings only
let str = "Hi, my name is "
let anotherString = "Pasan"
let myName = str + anotherString
// The following line of code won't work.
// Paste it in a playground and uncomment it.
// var concat = str + 2
/*
Swift uses string interpolation to include
the name of a constant or variable as a placeholder
in a longer string, and to prompt Swift to replace
it with the current value of that constant or variable.
*/
let name = "Pasan"
let nameString = "Hi, my name is \(name)"
// This also works with non string types
let temp = 52
let temperatureDisplay = "The current temperature is \(temp) Farenheit."
// You can also replace the evaluation of an expression using string interpolation
let celciusTempDisplay = "The current temperature is \((temp-30)/2) Celcius."
Hope this helps.
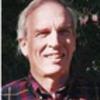
jcorum
71,830 Points"Concatenation allows you to combine to strings together and it only works on two strings."
An update. Not sure what earlier versions of Swift allowed, but currently you can concatenate more than 2 Strings together in the same statement:
let str = "Hi, my name is "
var concat = str + "2" + "3" + "4" + "5" + " works" //displays "Hi, my name is 2345 works"
Because both operands of + need to be Strings, you have to do a little extra work if you want to add a number to a String:
var concat2 = str + String(2) //displays "Hi, my name is 2"
Re why interpolation rather than concatenation, here's a quote from Apple's intro for interpolation: "String interpolation is a way to construct a new String value from a mix of constants, variables, literals, and expressions" In other words, you can use interpolation with numbers, booleans, etc. without first turning them into Strings, which you would have to do if you used concatenation.
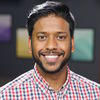
Pasan Premaratne
Treehouse Teacher"Concatenation allows you to combine to strings together and it only works on two strings."
That was meant to signify not the number of strings you can combine but that the types of both objects need to be the same. Thanks for pointing that out :)
In regards to the rest, you're correct but we explain the way we do so that we can abstract out some of the complexity. At this point (first Swift course), students don't know what initializers are so we stay away from String()
. String literals make it easy to introduce strings without having to point out that it is a struct and has properties.
Also using String()
doesn't really change the fact that you can only concatenate two String types. When you write String(2)
, what Swift does is call the description property on 2 (2.description
) which returns a String. We then use this string as an initializer argument to create a second String instance. With simple code the difference is negligible, but with a larger data set it may affect performance to make a second unnecessary call.
Using string interpolation calls the object's description property and uses that string directly. It's one less thing the compiler has to do basically.
Hope that makes sense. We avoid all this since it's only the first Swift language course
Stephen Hess
270 PointsStephen Hess
270 PointsSo is there ever a reason to use concatenation?
Pasan Premaratne
Treehouse TeacherPasan Premaratne
Treehouse TeacherWhen you're working with String instances only. In the code we're writing, it's not a big difference, however if you were combining hundreds of thousands of strings from a database, there might be performance gains with one over the other