Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial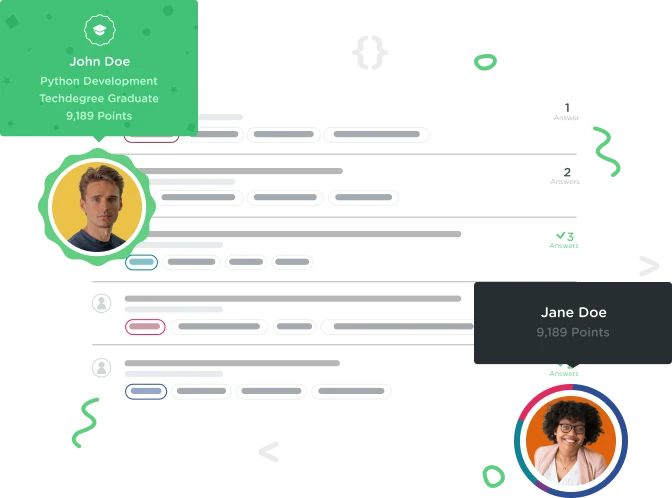

David Greenstein
6,734 PointsWhat is the difference between def add(*nums) and def add(base, *args)?
I understand they produce the same output but could someone help explain the difference. Right now def add(*nums) is making sense to me while im unsure what is the difference between base and args
3 Answers
William Li
Courses Plus Student 26,868 PointsHi David. I see that your question has not been answered in a satisfactory manner yet.
In python, the use of *args
or *nums
(the naming doesn't matter here) is a way to let function accept any number of arguments.
def add(*nums):
# this function accepts 0 or more arguments
# and the arguments are stored in a tuple named nums
result = 0
for i in nums:
result += i
return result
print(add()) # => 0
print(add(1,2,3)) # => 6
print(add(9)) # => 9
def add(base, *args)
on the other hand, accepts 1 or more arguments, base is the required argument here, any more argument provided after the 1st one will be stored in the tuple named args.
def add(base, *args):
# this function accepts 1 (minimum) or more arguments
result = base
for i in args:
result += i
return result
print(add()) # => syntax error (expected at least 1 argument, 0 given)
print(add(2)) # => 2
print(add(2,5,3)) # => 10
Does that make sense? Feel free to tag me if you would like more explanation on the subject. Cheers.

fahad lashari
7,693 PointsThanks!

youssef moustahib
2,303 PointsThat was great thanks, cleared it all up for me!

Hsieh Gardner
2,599 PointsWhy does the "def add(*num)" need to type in "result = 0" but the"def add(base,*args) doesn't ?

Lauritz Patterson
1,207 PointsWilliam Li this is a year later but this is SUCH a great explanation between the two. But why would you want to use one over the other. is there a benefit ? # def add(*nums): vs def add(base, *args):

Chapman Cheng
PHP Development Techdegree Graduate 46,936 Pointsthanks that's explain alot

David Greenstein
6,734 PointsThat doesnt answer the question? Taylor Espejo
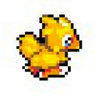
Taylor Espejo
3,939 PointsWhat david said
David Greenstein
6,734 PointsDavid Greenstein
6,734 Pointsdef add(base,*args): total = base for num in args: total += num return total add(5,20)
for reference