Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial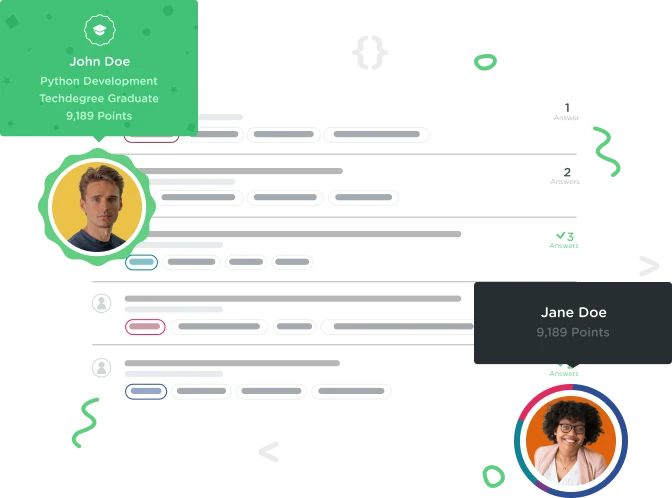

Ben Os
20,008 PointsWhat is the difference between for...of and for...each?
What is the difference between for...of and for...each in Javascript?
Take for example the following code:
for (const element of document.querySelectorAll(".elementor-button-link br")) {
element.style.display = "none";
}
//
document.querySelectorAll(".elementor-button-link br").forEach(e => e.style.display = "none");
The result will be similar. Both run on all nodes in the group (parent or/and children) but I still wonder how one would define a difference if there is any.
1 Answer
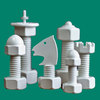
Steven Parker
231,269 PointsOne is a loop syntax of the language, the other is a method of a collection.
The "for...of
" syntax is a language statement used to construct a loop, and can be used with any iterable. The body of the loop will enclose statements that are to be repeated. Because it is a type of loop, you can use "break
" and "continue
" statements in the body.
But ".forEach()
" (not "for...each") is a method that you will find on certain kinds of collections. It can only be used on collections which define it (such as Arrays and nodeLists). It takes an argument which is a function to be called for each item. The function cannot contain "break
" or "continue
" statements (unless they appear inside an inner loop).
Ben Os
20,008 PointsBen Os
20,008 PointsI was honored to have your answer on this. You are a master of didactics. I hope you would one day teach here in Treehouse.
I have but one small, comment, I actually aimed to ask on the general difference between
for...of
andfor...each
, without relating it to Js but as I understand, the general difference is thatfor...of
is a most general type of loop, from which you can create any other loop, Like these four types:I mean, if I understand correct, for...of encapsulates these 4 types of loops.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsI wouldn't say "
for...of
" is a more general loop .. perhaps it's the opposite as it does not give you an index of the item. It works very well for objects but it would not be a good choice for other things, such as arrays. Each type of loop does its best job in some situations but not others.Ben Os
20,008 PointsBen Os
20,008 PointsSorry, I missed that part that in any given case it wont have an item-index. Please clarify what you mean by that it works very well for objects but not for arrays due that any array is an object.
Is the main difference than that
for...of
works for nodeLists and some other objects whilefor...each
works for both nodeLists, arrays, and some other objects?Steven Parker
231,269 PointsSteven Parker
231,269 PointsYes arrays are objects, but they are special in that the data is all in items with numeric keys. So a normal "
for
" loop is great since you can use the length property as the loop limit. A "for...of
" would not be as good with an array because it returns only the values of the items and not the indexes that identify them."
for...of
" can work with any object (including arrays), but ".forEach()
" (note: not "for...each") only works on objects where it has been defined as a method.Ben Os
20,008 PointsBen Os
20,008 PointsThanks Steven. I seem to miss only this: Why do you call the object's properties "non numerical"? I mean, length, as I see it, is numerical. For example, if the object or array has the properties/keys AA, BB, CC, their length is 0,1,2 which is numerical).
Ben Os
20,008 PointsBen Os
20,008 PointsThan, I assume that by "non-numerical property keys" you mean to "item references" (as when 0 is for the first property-value, or key-value, and so on, 1 is for the second, etc)?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThose are the numeric keys, the ones that contain the array data. I was talking about properties that had non-numeric keys.
Remember that "
for...of
" only gets the values of the items and not the keys. On the other hand, "for...in
" would get all the keys of the enumerable items, numeric or not, which you can then use to get the values.Ben Os
20,008 PointsBen Os
20,008 PointsFor feature readers, can you edit your comments to make sure there is no confusion (regarding for...in and for...of)?
Besides length, the numeric object property, what are the main non-numeric object properties? In a quick search I don't find an article that will simply list these.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsGood idea, comments fixed. And MDN discusses object properties including "length", "prototype" and "constructor". And if you include methods, that would extend the list a good bit more.