Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial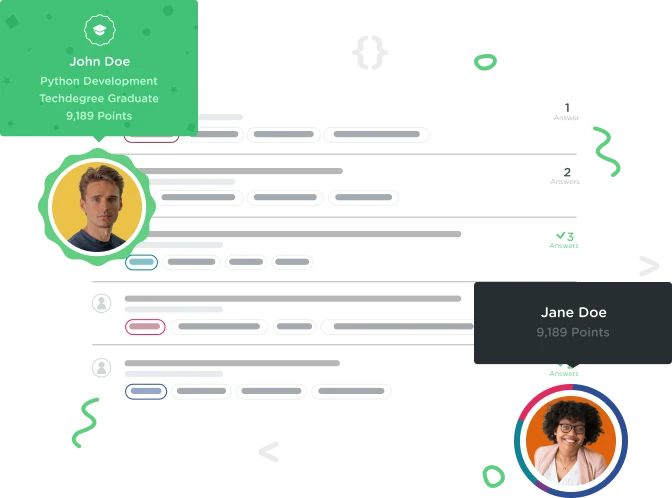
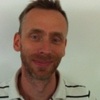
Magnus Rydberg
4,683 PointsWhat is the difference between key and index when we look at an associative array?
When I went trough the lesson lesson PHP Array Functions I found that I probably don't fully understand of how both associative arrays and indexes work.
I thought that when I create an associative array I actually replace the zero index system with a more or less arbitrary index made up of the strings I associate with the values.
I see that the output of var_dump(array_keys$names) indexes like so [0] => βMikeβ [1] => βChrisβ etc.
To me that indicates that a numbered index is still there even thouh I use stings as keys. It definitely makes sense to be able to number the associative array for any kind of organizing purposes.. But it also means that I might have misunderstood what an associative array is built from.
More specifically: How do I better understand the difference between index and key when we look at an associative array in particular?
2 Answers
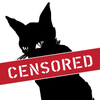
Patrizia Lutz
1,449 PointsAssociative arrays are differentiated by the fact that they have strings rather than numbers as index. But, they can be accessed the same way.
An numeric array could be created like this:
<?php
$numeric_array[0] = 1;
$numeric_array[1] = 2;
$numeric_array[2] = 3;
$numeric_array[3] = 4;
$numeric_array[4] = 5;
?>
Or, it could be created like this (more common):
<?php
$numeric_array = array( 1, 2, 3, 4, 5);
?>
You can access the second value in the array using the index 1
,
<?php
echo $numeric_array[1];
//=>2
?>
Each item can be output using a foreach
loop, like this:
<?php
foreach( $numeric_array as $value ) {
echo "Value is $value <br />";
}
//=>Value is 1
//=>Value is 2
//=>Value is 3
//=>Value is 4
//=>Value is 5
?>
You can create an associative array like this
<?php
$associative_array["apple"] = 1;
$associative_array["orange"] = 2;
$associative_array["banana"] = 3;
?>
Or, you could create it like this:
<?php
$associative_array = array(
"apple" => 1,
"orange" => 2,
"banana" => 3
);
?>
The value for the key orange
can be output this way:
<?php
echo $associative_array["orange"];
//=>2
?>
To simply get the values, you can loop through the array in the same way as you would a numeric array, like this:
<?php
foreach( $associative_array as $value ) {
echo "Value is $value <br />";
}
//=>Value is 1
//=>Value is 2
//=>Value is 3
?>
To get the keys and values, you can loop through them like this
<?php
foreach( $associative_array as $key => $value ) {
echo "Value of $key is $value <br />";
}
//=>Value of apple is 1
//=>Value of orange is 2
//=>Value of banana is 3
?>
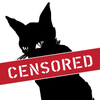
Patrizia Lutz
1,449 PointsYou can use this to get the nth element in the array:
$newArray = array_keys($associative_array);
echo '$newArray[1] is: ' . $newArray[1];
//=>$newArray[1] is: orange
Note: since indexes starts at 0 (not 1), you should use 99 to get the 100th item,
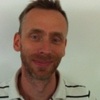
Magnus Rydberg
4,683 PointsThanks again Patrizia, that's very helpful.
Magnus Rydberg
4,683 PointsMagnus Rydberg
4,683 PointsHello Patrizia
Thank you very much for your detailed answer.
So far I understand that any regular array I declare will by default have a zero based index.
In the associative array I replace the zero based index with the string keys I create. The zero based index is then lost.
Let's say I have an enormous associative array and I want to find for example the 100th key. Is there still a way for me to do so?