Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial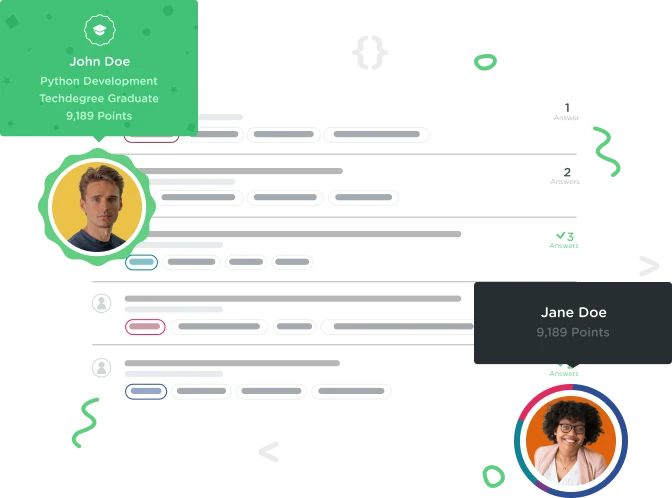

Jiten Mistry
4,698 Pointswhat is the difference between passing by value and passing by reference?
what is the difference between passing by value and passing by reference? if someone could give an example, it would much appreciated.
thank you
2 Answers
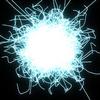
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Jiten,
The main difference is when we pass by reference we are handing in our only copy of the variable, and the function directly modifies that variable, where as when we pass by value we have another variable that points at the other variable, so basically two copies of the same variable, and only the copy is modified leaving our original unchanged.
Think of the GoKart examples that we have worked with previously, and consider this function, I'll try to comment my code. to keep it up, let's say we have two different variables and are writing another charge function.
public int mBarsCount = 8;
// this is the only variable we have of this function and it is directly modified by our function, this is passing by reference.
public int drive(int laps) {
mBarsCount -= laps;
return mBarsCount
}
Here is the example of passing by value.
public int mBarsCount = 8;
public int currentBarCount = mBarsCount;
// we are assigning two variables in this.
public int drive(int laps) {
currentBarCount -= laps; // in our function we only decrease the copy, never modifying our original variable mBarsCount.
}
In essence, when we are modifying our variable directly we are passing it by reference, when we are creating a copy of our variable and modifying the copy only and keeping the original the same we are passing by value.
Think of passing by value as hand writing an essay. That is your only true copy, you then photo copy 10 sheets of it and let it be passed around, you are now passing your variable the essay, as value.
Thanks I hope this helps, shout at me if it doesn't.

Craig Dennis
Treehouse TeacherHi Jiten!
The good news is Java is only pass by value. What this means is that when a method is called the arguments passed in are copied, so changing it in the method won't affect things in the outer scope.
Programming languages like C and C# allow you to specify that they are modifiable, but Java does not.
Confusion arises when object references are used as arguments. The reference is still copied, but because the reference is pointing to the same object, it seems like it is changing the argument, but really its just using a copy of the reference.
Hope that makes sense.
tl;dr. Java is only pass by value.