Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial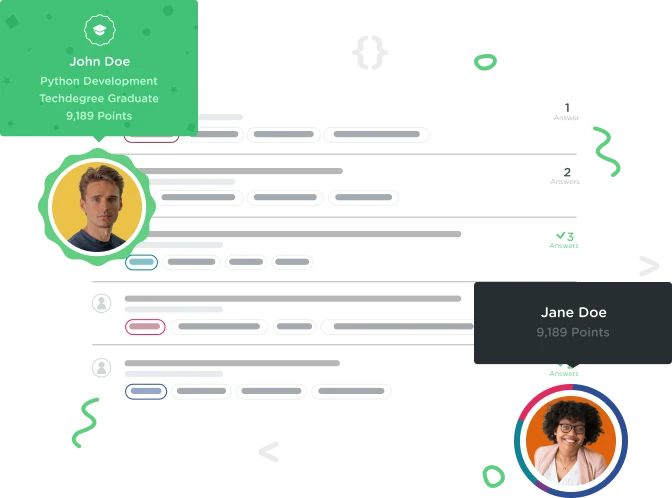

Felix Putra
Python Web Development Techdegree Student 1,927 PointsWhat is the difference between printf and println? And what does the term "console" actually mean?
Hi treehousers,
I have been learning java with treehouse for the past few days and I'm confused about the difference between "printf" and "println". Furthermore, I also don't know what does the term "console" mean and what it is for? sometimes people use "console.printf" and "System.out.printf". What are they?
Thanks before!
3 Answers
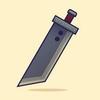
Allan Clark
10,810 PointsFirst for the difference in println and printf. The names are short versions of their function. println is short for "print line", meaning after the argument is printed then goes to the next line. printf is short for print formatter, it gives you the ability to mark where in the String variables will go and pass in those variables with it. This saves from having to do a long String concatenation. Here are a few examples, I will be putting '_' where the computer is left and where it would put stuff if sent another print statement.
Base print statement:
System.out.print("What do you mean Darth Vader's my father?!?");
Result printed to the User in the console:
What do you mean Darth Vader's my father?!?_
println statement:
System.out.println("What do you mean Darth Vader's my father?!?");
Result printed to the User in the console:
What do you mean Darth Vader's my father?!?
_
printf statment:
good resource: http://alvinalexander.com/programming/printf-format-cheat-sheet
System.out.printf("What do you mean %s is my father?!?", dad);
What do you mean Darth Vader's my father?!?_
*side note: this assumes there is a String variable names dad = Darth Vader's
As for console vs System.out: In general the console is like (and can be) the command prompt in Windows very basic text input/output.
System.out is telling Java that you want to use the output steam to the console.
The word console is used in code basically as a wrapper for System.out. Some TreeHouse challenges have code that is abstracted away from you. This includes the declaration of a console variable. IIRC the declaration will have System.out in it.
Hope this helps, let me know if I can clear anything up.
May the Java force be with you
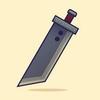
Allan Clark
10,810 PointsSystem.out is the raw output stream Object for the JVM. You can think of this stream as a conveyer belt of text to the user. At the user end of the conveyer belt it displays the text to whatever console is associated with the JVM (i.e. Windows cmd, Mac terminal, IDE console etc).
Of course we have to receive input from the user as well. System.in is the raw input stream from the console. In the metaphor this will be a conveyer belt going in the opposite direction.
The Console Object in code is like a String processing machine at our end of the input/output stream conveyer belts. It gives us more advanced options when doing input/output, as well as combining both streams into one Object.
A good illustration for this would be on the input side. With the Console Object we get a readPassword() method. This will get a String from the user while not displaying the characters the user is typing. If we tried getting the password directly from the input stream System.in we would have no way of hiding what the user is typing.
Unfortunately I can't come up with a good illustration of the benefit of Console for the output stream, cause they are both just putting text on a screen. In the coding real world it will be better to use Console (or similar Object) if you will be doing much user input/output. I really only use System.out for quick one time outputs that don't need any fancy-ness with it, such as debugging.
System.out.print("bro your code gets this far without breaking");
https://docs.oracle.com/javase/7/docs/api/java/lang/System.html https://docs.oracle.com/javase/7/docs/api/java/io/Console.html

Kyle Nowack
185 PointsCan console be used the same way as System.out.print ? I am taking a java class in college now and the teacher only has knowledge on System.out.print. If I were to replace all my System.out.print with console.print will my code still work?
Felix Putra
Python Web Development Techdegree Student 1,927 PointsFelix Putra
Python Web Development Techdegree Student 1,927 PointsHey thank you so much! I'm much clearer about the printf and println. However, I'm still yet not understand the console and the System.out. Mind if you give me an example? Thanks!