Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial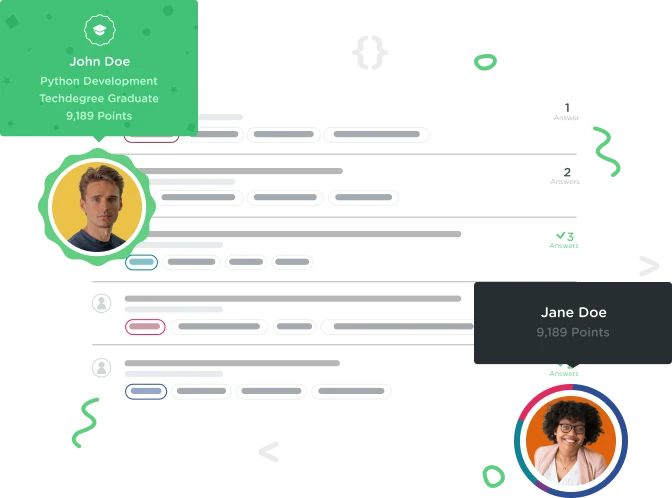

Gustavo Barrera
704 Pointswhat is the difference between print_r() and echo? are they the same ?
Associative arrays
2 Answers
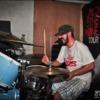
Mike Costa
Courses Plus Student 26,362 Pointsecho and print_r are 2 different beasts. echo is a language construct that can "act" as a function, and by act, I mean you can echo 1 string if its within parenthesis. These are valid.
echo ("Hello");
echo "Hello", "world";
However, this is not:
echo ("hello", "world");
echo, i believe, will only output data types of string, int, bool, float. It will not output arrays, or objects. If you try to echo an array, it will output Array with no details to it.
print_r is a function that will format the variable that you pass into it. So for instance, if you have an array, it will format it to a human readable output.
$var = array(1,2,3,4);
print_r($var);
// Outputs:
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
)
But you can also store the results of print_r into a variable with a second param to print_r, which will prevent any output from the function.
$var = array(1,2,3,4);
$output = print_r($var, true);
echo $output; // outputs the returned string from print_r
var_dump is another function that goes into slightly more detail that print_r, where it will output the the data type and values of what you're passing into it
$var = array(1,2,3,4);
var_dump($var);
// Outputs:
array(4) {
[0]=>
int(1)
[1]=>
int(2)
[2]=>
int(3)
[3]=>
int(4)
}
$string = "Hello World!";
var_dump($string);
// Outputs:
string(12) "Hello World!"
I hope this helps clarify the similarities and differences between echo, print_r, and var_dump.
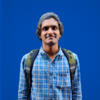
MELVIN GEORGE
3,490 Pointsthanks