Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial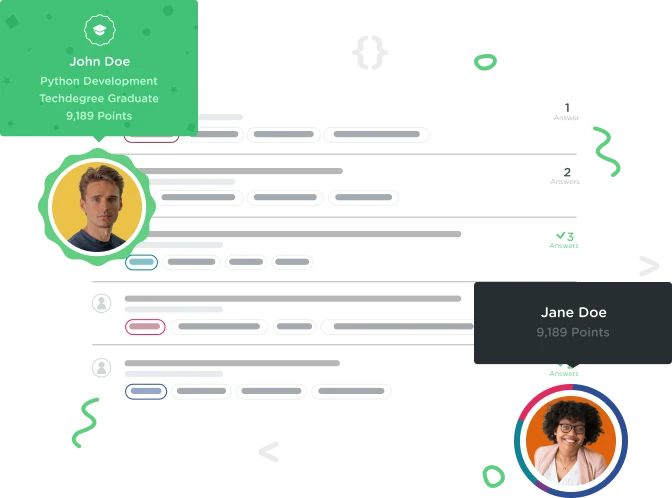

Bogere Sanders
2,195 PointsWhat is the difference between puts vs return when defining a method?
im having trouble distinguishing the difference
2 Answers

Daniel Cunningham
21,109 PointsI struggled with that in the beginning as well. When you're typing the values into IRB or a console, the return and the puts or even print methods look like they do the same thing because all of them appear to be output into a readable format. But they are very different in nature.
Lets say you had a method that added two strings together. Call it anything, but I'll call it "addstring". Code it like this: <br>
def addstring(x,y)
z = x + y
return z
end
<br> Then make another method to add two strings. i'll call it "putstring" and code it like this:
def putstring(x,y)
z = x + y
puts z
end
Now lets say we want to make a sentence "Have Fun Today" and we want to add it one word at a time (because nothing is going to be easy) and we want to store the value in variable 'words'. We need to use the above functions to make it. The first function "addstring" could be used like this:
words = addstring(addstring("Have ","Fun "),"Today")
Calling the variable will return "Have Fun Today"... So it works and that's great. Now lets do that with putstring
words = putstring(putstring("Have ","Fun "),"Today")
This function will fail miserably and produce a "NoMethodError" with the warning "undefined method for + in NilClass".
This happens because a return value is something that is "returned" from a function, and as a result, it can be stored into an object and used (or reused) in a function. puts on the other hand will return NOTHING but it will 'print' the value to the screen. As a result, puts will not be stored and will, in fact, return a nil value.
Long story short (too late), return gives you the ability to reuse values in other functions, while puts will merely print it to the screen. Definitely try these methods yourself in IRB and attempt to store 'puts' values into variables. You'll see the different responses and get a better idea on how these methods work.
Hope that helps!
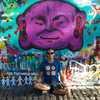
Andrew Stelmach
12,583 Pointsputs prints whatever is after it and THEN RETURNS 'NIL'.
'return', on its own, returns 'nil'. However, you can put something after return.
A few examples methods, showing what they return when called:
def method1
return
array = []
end
def method2
array=[]
end
def method3
array=[]
return
end
def method4
puts "Hello"
end
def method5
x = 2
something_random = "something_random"
end
def method6
x = 2
something_random = "something random"
return x
end
method1()
=> nil
method2()
=> []
method3()
=> nil
method4()
Hello
=> nil
method5()
=> "something random"
method6()
=> 2
Remember, it's important to play around in irb to experiment.
Patrick Montalto
7,868 PointsPatrick Montalto
7,868 PointsYou can generally leave out the return method in most Ruby functions. A Ruby function will automatically return the last thing evaluated, unless an explicit return comes before it.