Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial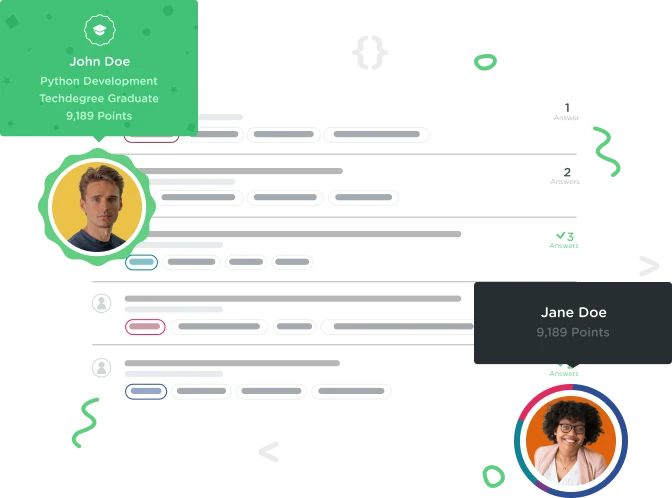

peter keves
6,854 PointsWhat is the difference between Raw Values and Associated Values ?
What is the difference between Raw Values and Associated Values ? I have watched both videos but still don't understand the difference can somebody help me with this please ?
2 Answers
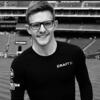
agreatdaytocode
24,757 PointsHello Peter,
Maybe this will help:
Raw values are for when every case in the enumeration is represented by a compile-time-set value. The are akin to constants, i.e.
let A = 0 let B = 1 is similar to:
enum E: Int {
case A // if you don't specify, IntegerLiteralConvertible-based enums start at 0
case B
}
So, A has a fixed raw value of 0, B of 1 etc set at compile time. They all have to be the same type (the type of the raw value is for the whole enum, not each individual case). They can only be literal-convertible strings, characters or numbers. And they all have to be distinct (no two enums can have the same raw value).
Associated values are more like variables, associated with one of the enumeration cases:
enum E {
case A(Int)
case B
case C(String)
}
Here, A now has an associated Int that can hold any integer value. B on the other hand, has no associated value. And C has an associated String. Associated types can be of any type, not just strings or numbers.
Any given value of type E will only ever hold one of the associated types, i.e. either an Int if the enum is an A, or a String if the enum is a C. It only needs enough space for the bigger of the two. Types like this are sometimes referred to as "discriminated unions" – a union being a variable that can hold multiple different types, but you know (from the enum case) which one it is holding.
They can even be generic. The most common example of which is Optional, which is defined like this:
enum Optional<T> {
case .Some(T)
case .None
}
From stackoverflow

peter keves
6,854 PointsThanks :)))