Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial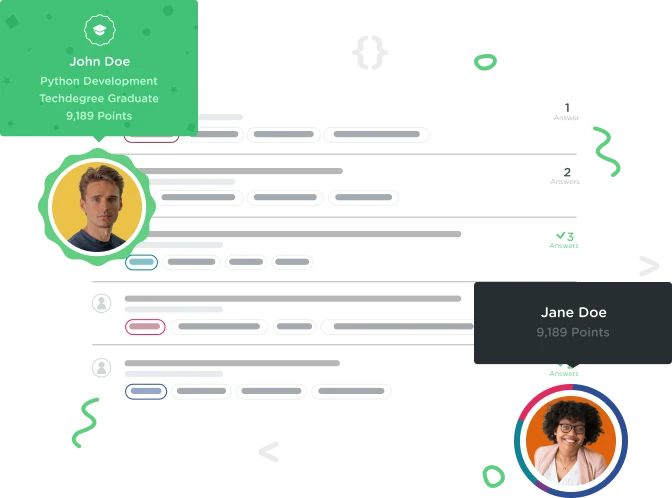

gabriel wambua
5,061 PointsWhat is the difference between setters getters and constructors?
Hi Team,
Please help me understand the difference between setters, getters and constructors and when they can be used.
2 Answers

Joe Rimuka
5,823 Pointsconstructor is a method which is called when object of the class is instantiated. It is used to set default values to the attributes of the class.The name of the constructor is the same as the class name. Getters and Setters are methods that are used to access the attributes of an object. The attributes are mostly kept private so we cannot access them directly so the getter and setter are used to access them. These methods are mostly public but never private. hope it helps
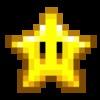
Tom Achki
3,680 Points- Constructors are used when we create an object. It is used to provide some information about the object. For example, if I create book object, I can prompt for extra information about it in the constructor, such as color and number of pages. Constructor allows you to do that.
class Book {
private String color;
private int numberOfPages;
//Constructor
public Book(String color, int numberOfPages){
this.color = color;
this.numberOfPages = numberOfPages;
}
//Getter for color
publc String getColor(){
return color;
}
//Getter for numberOfPages
publc int getNumberOfPages(){
return numberOfPages;
}
//Setter for color
public void setColor(String color)
this.color = color;
}
}
When I create Book object, I should specify color and number of pages, as we required it in the constructor.
Book book = new Book("blue", 120);
- Getters are used to get the value of private variable. For example, color and numberOfPages variables are private, so I can not access it outside the class. Getters allow us to do that. And setters allow us to change their value.
System.out.printf("The %s has %d pages", book.getColor(), book.getNumberOfPages()); // Using getters to access private variables
- You can imagine changeColor() method.
public void changeColor(){
book.setColor("red");
}
So this method changes color of the book using the setter. So setters are used when we need to change the value of a private variable.