Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial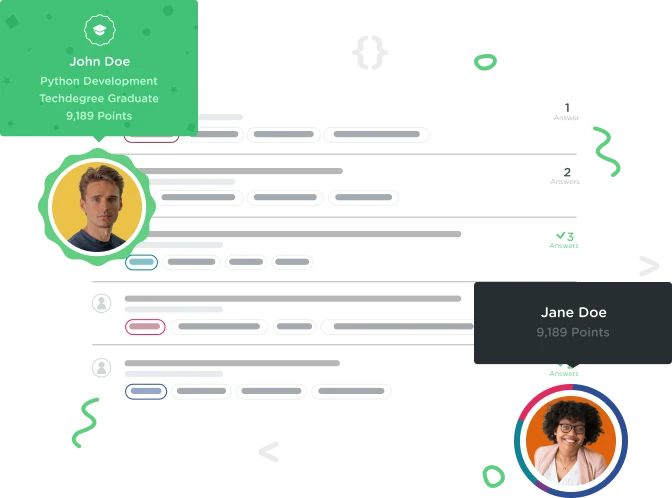
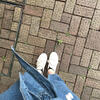
Tani Huang
6,952 PointsWhat is the different using 'single quote' and "double quote" ?
//double quote work fine (javascript)
document.querySelector("#index");//ok
//double quote work fine (javascript)
for (let i = 0 ; i < list.length ; i += 1) {
list[i].addEventListener ("mouseover", () => {//ok
list[i].style.backgroundColor = "red";
});
list[i].addEventListener ("mouseout", () => {//ok
list[i].style.backgroundColor = "white";
});
}
//double quote error (jquery)
var url = "../data/employees.json";//json data
$.getJSON (url,function(response) {
var statusHTML = "<ul class="bulleted">";//error
$.each(response,function(index,employee){
if (employee.inoffice === true) {
statusHTML += "<li class="in">";//error
} else {
statusHTML += "<li class="out">";//error
}
statusHTML += employee.name + "</li>";//ok
});
statusHTML += "</ul>";//ok
$("#employeeList").html(statusHTML);
});
2 Answers
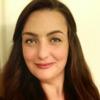
Jennifer Nordell
Treehouse TeacherHi there! The key is that either double quotes or single quotes both begin and end a string. This presents a problem when we actually need a double quote or single quote as part of our string.
For instance, if we wanted to print a famous quote to the page with the double quotes as part of our string:
document.write('Winston Churchill once said: "Success consists of going from failure to failure without loss of enthusiasm" ');
We need the single quotes here so that JavaScript understands that in this case, the double quotes are not the beginning of a string nor the end. They are part of our string. The same thing is true for apostrophes (single quotes). If you need an apostrophe in your string, then you need to either start the string with a double quote or escape the apostrophe.
In this case, where you're seeing errors is because the HTML we're writing needs to say something like:
<li class="in"></li>
That class name must be in double quotes in the HTML for it to work. So we start that string with single quotes so that it understands that the double quotes are part of the string.
Hope this clarifies things!
edited for correction
I always thought that the class had to be in double quotes. I stand corrected. But the premise of my statement still stands. Mixing quotes gets complicated when you need a quote to be part of a string.
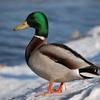
Robert Schaap
19,836 PointsThere's no real difference between using one or the other, but using them mixed can be a bit tricky. In your case for example, "<li class="in">"
is a piece of HTML you're storing into a variable. The pair of quotes right before the "in" word, tells the compiler that the string has ended. Next is the word in, by itself and then another string. Basically because you're trying to put a string into a string in this way, and are using the same quotes, it breaks.
You have a few options:
"<li class=\"out\">" // escape the quotes to be ignored with \.
"<li class='out'>" // use different quotes on the class name.
'<li class="out">' // use different quotes on the piece of html.
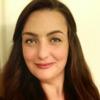
Jennifer Nordell
Treehouse TeacherI've changed your "comment" to an answer to allow for voting Thanks for helping out in the Community!
Robert Schaap
19,836 PointsRobert Schaap
19,836 PointsThat last comment isn't completely true. The standard is to use double-quotes for attributes in HTML, but it actually doesn't matter. If you want to write
class='test'
andid="test"
on the same line, it will work. The browser will interpret the file just fine, but it will clean up all the quotes to double quotes. As long as you're not mixing quotes, you're fine.See this horrible js fiddle for an example. Just don't judge me. https://jsfiddle.net/6r3bj54z/
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherRobert Schaap I stand corrected. For some reason, I thought they must be in double quotes.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherAlso, Robert brings up an excellent point in his jsfiddle. You can avoid this scenario entirely by using JavaScript Template Literals. Treehouse has an excellent 9 minute workshop on them here. Learning them will save you tons of concatenation headaches down the road