Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial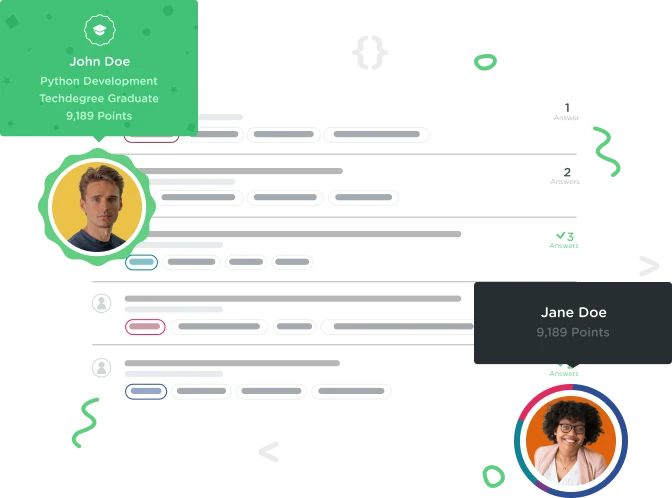
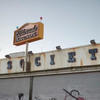
Vic A
5,452 Pointswhat is the issue here? My code looks correct to me.
For some reason this code isn't working quite right. Need help
def disemvowel(word):
word = list(word)
vowels = list("aeiouAIEOU")
for v in word:
if v in vowels:
word.remove(v)
else:
continue
word2 = ''.join(word)
return word2
2 Answers
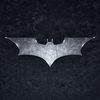
Logan R
22,989 PointsYour solution basically works but you have a small problem. You are modifying your variable word
in the middle of the loop with your .remove(v)
. This messes up the loop and doesn't properly iterate over it.
Some examples:
> disemvowel("HellOoO")
'Hllo'
> disemvowel("HelloO")
'HllO'
A simple solution that works in this case is to just iterate over a copy of the list. This way as you modify the list, it doesn't modify the list it is iterating over. To do this, you can just do:
for v in list(word):
.....
If you can't get it to work, feel free to leave a reply!
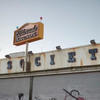
Vic A
5,452 PointsThanks a lot for the help. Although I don't fully understand the fix, your solution fixed the problem. Also, I am curious to know if you know the cleanest/shortest way to write this function. I feel there is a more concise way than I have attempted.
Thanks Again
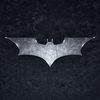
Logan R
22,989 PointsCheck out Caleb Shook reply above. He explains why pretty well.
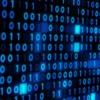
Alexander Davison
65,469 PointsThe shortest possible way is to jam up the whole thing onto two lines.
def disemvowel(word):
return ''.join([letter for letter in word if letter.lower() not in 'aeiou'])
The shortest and cleanest way to write it is to expand the previous example. This is my preferred way of tackling this challenge.
def disemvowel(word):
result = ''
for letter in word:
if letter.lower() not in 'aeiou':
result += letter
return result
I hope this helps
~Alex
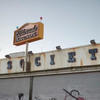
Vic A
5,452 PointsAwesome. Thanks man. I didn't know you could iterate through string using 'not in'. Now I know.
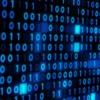
Alexander Davison
65,469 PointsNo problem :)
You can also use in
and not in
on lists.
Caleb Shook
3,194 PointsCaleb Shook
3,194 PointsI was running into the same issue. When altering lists with a loop, I learned it is best to work with a copy of the list. When you call the remove() function, after removing the value, the rest of the indexes get decremented. For example; you call disemvowel on "aAc" you would get back Ac because it skipped over 'A' due to its index moving backward. You should create a copy of the list before the for loop. Then iterate through the original list and remove the vowels from the list copy and return that one.