Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial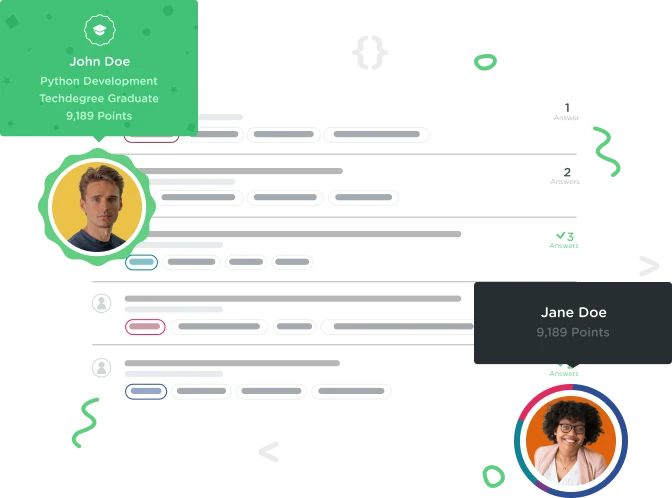

Akshat Gupta
1,438 Pointswhat is the logical difference between a js object and js map as both are a collection of key value pairs?
JS maps and JS objects are both collection of key value pairs and seem almost same logically so while writing code when would be a map be beneficial as compared to an Object
2 Answers

Christian Andersson
8,712 PointsThere are many similarities indeed and not too much differentiate the two. I think the biggest benefit in using map
is that it comes with some default features that you might find useful.
This includes iterators which allow you to traverse through the items in the map. It also provides certain methods, such as clear()
, set()
and so on.
The MDN has a nice explanation:
Objects are similar to Maps in that both let you set keys to values, retrieve those values, delete keys, and detect whether something is stored at a key. Because of this (and because there were no built-in alternatives), Objects have been used as Maps historically; however, there are important differences between Objects and Maps that make using a Map better:
- An Object has a prototype, so there are default keys in the map. This could be bypassed by using map = Object.create(null) since ES5, but was seldomly done.
- The keys of an Object are Strings and Symbols, where they can be any value for a Map.
- You can get the size of a Map easily while you have to manually keep track of size for an Object.
This does not mean you should use Maps everywhere, objects still are used in most cases. Map instances are only useful for collections, and you should consider adapting your code where you have previously used objects for such. Objects shall be used as records, with fields and methods. If you're still not sure which one to use, ask yourself the following questions:
- Are keys usually unknown until run time, do you need to look them up dynamically?
- Do all values have the same type, and can be used interchangeably? -Do you need keys that aren't strings? -Are key-value pairs often added or removed? -Do you have an arbitrary (easily changing) amount of key-value pairs?
- Is the collection iterated?
Those all are signs that you want a Map for a collection. If in contrast you have a fixed amount of keys, operate on them individually, and distinguish between their usage, then you want an object.

Todd MacIntyre
12,248 PointsAlso, do note that a map is still technically an Object and inherits from Object.
map = new Map();
typeof map; // returns "object"
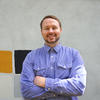
Tom Geraghty
24,174 PointsAlso iterating over Maps is almost always faster than just using a regular ol' object. See https://jsperf.com/es6-map-vs-object-properties/99
Akshat Gupta
1,438 PointsAkshat Gupta
1,438 PointsThanks Christian for this clear distinction between the two, this is what i was looking for. This answer made things a lot clearer
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsGlad I could help :)