Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial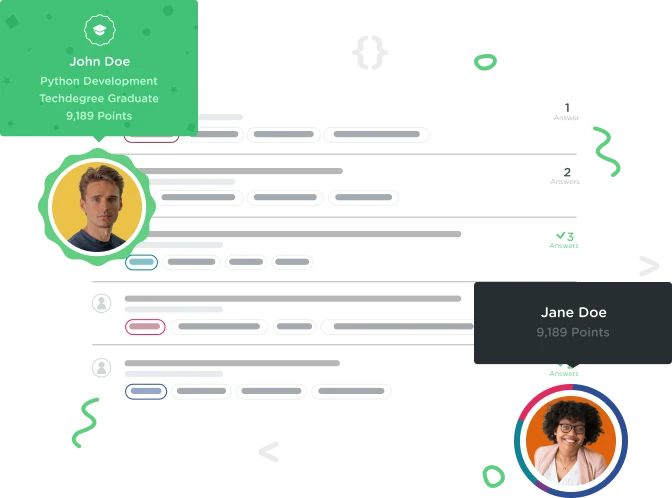

bhaktipriya shridhar
2,350 Pointswhat is the most "pythonic" way to read 2d arrays from input?
how do we use 2 for loops to read input in python? Also, is there a more compact way to read things from the input? list comprehensions maybe?
2 Answers

Mikolaj Magnuski
2,675 PointsI'd say it depends on what you mean by input (how it's structured etc.).
If it is a file with a clear structure (tab-delimited, csv, etc.) and homogeneous data type (for example all floats) I'd go with numpy
:
import numpy as np
data = np.genfromtxt('some_file.csv', delimiter=',')
The data in the example above is a naumpy array and could look like this:
array([[ 12., 45., 21., 30.],
[ 11., 22., 16., 35.],
[ 39., 29., 28., 41.]])
Numpy arrays are fast and easy to operate with. If your input is 2d you can index specific rows and columns in the following way:
# get the first row
data[0, :]
# returns:
array([ 12., 45., 21., 30.])
# get the second column
data[:, 1]
# returns:
array([ 45., 22., 29.])
# get the value at intersection of third row and second column:
data[2, 1]
# returns:
29.0
Depending on your needs you could also try pandas
(or many other ways including simple loops or list comprehensions).

Iain Simmons
Treehouse Moderator 32,305 PointsYou'd have to ensure the string was entered in a particular way, say, with comma separated items in each inner array and semicolons separating the arrays within the outer array. e.g.
string = '0 0, 0 1, 0 2; 1 0, 1 1, 1 2; 2 0, 2 1, 2 2'
Then you could use a list comprehension to split
the strings (note the space after the comma/semicolon):
array_from_string = [s.split(', ') for s in string.split('; ')]
Alternatively, you could use either ast.literal_eval or json.loads if the input is either written as valid Python lists or JSON arrays respectively.
That being said, I'd go with the suggestion of Mikolaj Magnuski and use numpy as it looks very useful.