Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial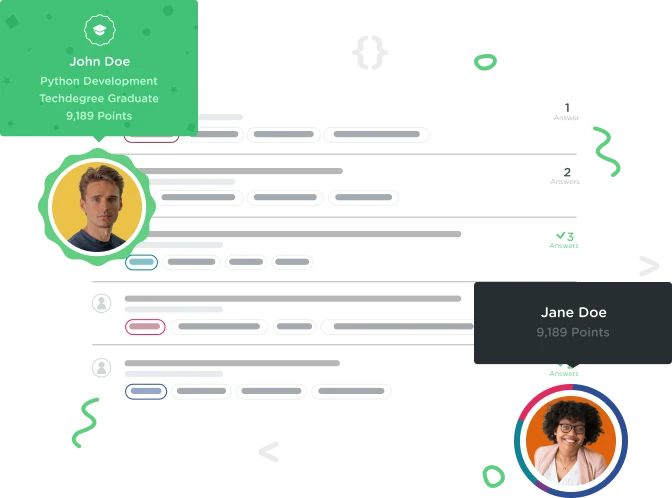

Youssef Moustahib
7,779 Pointswhat is the need for an argument in the function?
for examples in the refractor question, the function "show_list" has an argument of the list shopping_list. The code works perfectly well without the argument too though. I haven't finished the code challenge here but tried it in workspaces, it still works fine without the arguments?
Am I missing something here?
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
2 Answers

Unsubscribed User
9,496 Points# make a list to hold onto our items
shopping_list = []
It works because the name of the list in the actual program is also called 'shopping_list.' So when you remove the arguments from the function, the function uses the global 'shopping_list' created down below. With an argument, you can pass in any list, regardless of it's name. Try removing the arguments, like you've done, then change the line shopping_list = [] to s_list = [], and it will no longer work.
you can also keep the arguments and tweak the code like this, and it will work:
# make a list to hold onto our items
s_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(s_list)
continue
add_to_list(s_list, new_item)
show_list(s_list)
the above would not work without the argument. I hope that makes sense.
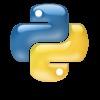
Viraj Deshaval
4,874 PointsThis works because shipping_list = [] is in global scope so any function can view it. If you place this in some function it will not be referenced by other functions. This means that it will be referenced locally by that function.
Youssef Moustahib
7,779 PointsYoussef Moustahib
7,779 PointsHi Colby,
Thanks for your response, but I'm still struggling to understand it, could you break it down a bit further for me please?
Unsubscribed User
9,496 PointsUnsubscribed User
9,496 Pointssure,
so down below, where it says:
That line creates a list called "shopping_list" that has a global scope, which means that it's accessible to any function created within the file. It just so happens that the name of the argument show_list and add_to_list is also called "shopping_list." The shopping_list that is worked with inside those functions is the argument, or the list that gets passed in as an argument. However, because they are named shopping_list, and you have a global shopping_list, the functions, if you remove the arguments, it will still work because you have that global variable 'shopping_list.'
Now, if you change the global variable from 'shopping_list' to 'things_to_get' and pass it through those functions, it will still work, because it is using the argument to work with the function. However, if you remove the arguments, it won't work, because they will be looking for a list called "shopping_list" that no longer exists.
Let me know if you need any further clarification, and I'll try and help more.
Youssef Moustahib
7,779 PointsYoussef Moustahib
7,779 PointsHi Colby,
Thanks again for the response, could you clarify the 2nd paragraph for me please.
I understand that a global variable can be used by all functions, I changed the name of the variable but I'm not sure what you meant by passing it through the function? Why would they still be looking for 'shopping_list' if you have changed it to 'things_to_get'? Isn't that just the exact same thing as having the 'shopping_list' variable?
Could you change the code and post it for me, like a before and after so I can see what you mean?
Thanks again