Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial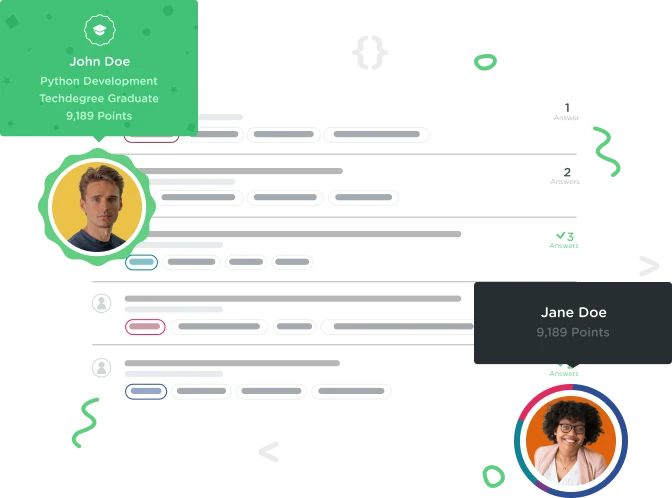

Ivan Diaz
634 PointsWhat is the new key word?
I can solve this problem, when i click in the check work button it send me a message that says Please create a new GoKart with the new key word. Please help me
public class Example {
public class GoKart{
private String mColor;
public GoKart(String getGoKart){
mColor = getGoKart;
}
public String carColor(){
return mColor;
}
}
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
}
}
6 Answers
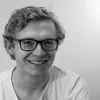
Luke Glazebrook
13,564 PointsTo instantiate (create a new instance of) an object in Java you will need to use the following syntax.
ClassName yourObjectName = new ClassName()
So, to create a new GoKart you could do the following!
GoKart newGoKart = new GoKart()
I hope that this helps!
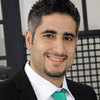
Ahmad El Masri
13,003 PointsI think you should declare the new "GoKart" in the main method;
Like that:
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart gokart = new GoKart("red");
}
}
I hope that this helps!

Ivan Diaz
634 PointsI have already added the new keyword and all of that but it keeps sendind me the same error here is the code:
public class Example {
public static class GoKart{ private String mColor;
public GoKart(String karColor){
mColor = karColor;
}
public String getKartColor(){
return mColor;
}
}
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart newGoKart = new GoKart(" New Red");
System.out.printf("The newGoKart color is %s\n",
newGoKart.getKartColor());
}
}

Ivan Diaz
634 Pointspublic class Example {
public static class GoKart{
private String mColor;
public GoKart(String karColor){
mColor = karColor;
}
public String getKartColor(){
return mColor;
}
}
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart newGoKart = new GoKart(" New Red");
System.out.printf("The newGoKart color is %s\n",
newGoKart.getKartColor());
}
}

Craig Dennis
Treehouse TeacherJust work in the main method, looks like you embedded the GoKart
class. You don't need it in this file.
Sorry for the confusion!

Frederick Reiss
15,003 PointsThank you Craig, https://teamtreehouse.com/library/java-objects-retired/meet-objects/creating-new-objects
This challenge was the most difficult for me. But it maybe more true to life, because the stated requirements and or previous challenge requirements we as developers will always have to deal with it.
I included the challenge code that worked for me... Thanks, -fred
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart color = new GoKart("red");
System.out.printf("The color of the GoKart is %s\n",
color.getColor());
}
/*
public class GoKart {
private String mColor = "red";
//GoKart gokart = new GoKart(mColor); // GoKart object
public GoKart(String color) { mColor = color; }
public String getColor() { return mColor; } } */ }