Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial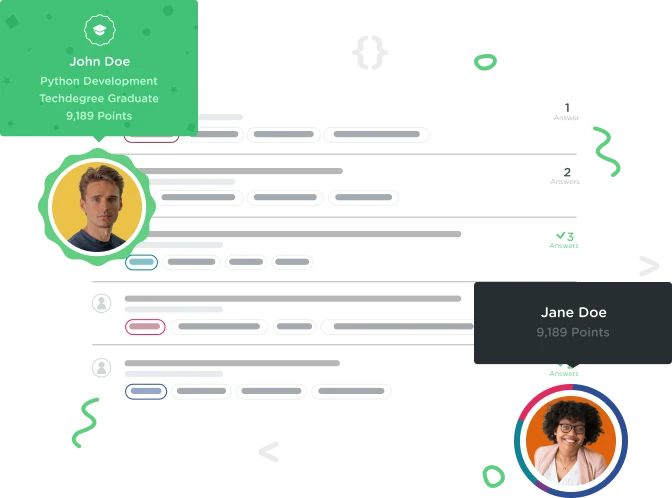

Pratham Patel
4,976 PointsWhat is the point of learning object oriented programming
In the java objects course we model apes dispenser We take two files Why couldn't we just do it on one file instead
1 Answer

Derek Markman
16,291 PointsWith smaller java projects you could very well just put all of your code into one file. The reason behind using multiple files, besides just good habit/practice, is so that if you were creating a larger project with a lot of code and extra functionality, your one file with the "main method" doesn't get cluttered up and your code is easier to understand.
If this is confusing I can try to explain it in a different way.
Pratham Patel
4,976 PointsPratham Patel
4,976 Pointslike your answer. When I first started object oriented programming I thought only about objects when the course teaches you the language such as methods and classes. But if you wouldn't mind I would like you to explain it in a different way
Derek Markman
16,291 PointsDerek Markman
16,291 PointsHere, let me try and give you an example and let's see if that helps you out a bit more.
Say we're making a hangman game. We would need like 3 main components: The main class(This class will only hold our main method, which is the entry point for a pure java source project), A Prompter class(This class will do the prompting to the user to guess the letter and what not), and finally a Hangman logic class(This class will hold the logic that actually makes the game playable after the user is prompted for a letter).
Technically, like I said in my previous post, we can put all 3 main components inside just one file and call it Hangman.java or something, but instead we would put each of the 3 main components in their own respective classes, so the one file that holds the main method doesn't get cluttered up.
In this example don't worry about the names of the methods etc... This is just to give you an idea of what I was talking about earlier.
Main.java
Prompter.java
HangmanLogic.java
In all of these classes just imaging instead of blank methods that we had tons of code. You can already see how it would make our files less cluttered and much easier to follow along with and read, if you wanted to say add additional functionality to our hangman game.
Let me know if this makes things any less confusing for you as to why it's beneficial to put your logic into separate classes instead of just one huge one. :)
Pratham Patel
4,976 PointsPratham Patel
4,976 PointsThanks it really cleared it up
Derek Markman
16,291 PointsDerek Markman
16,291 PointsNo problem, glad to help. Happy coding :D