Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial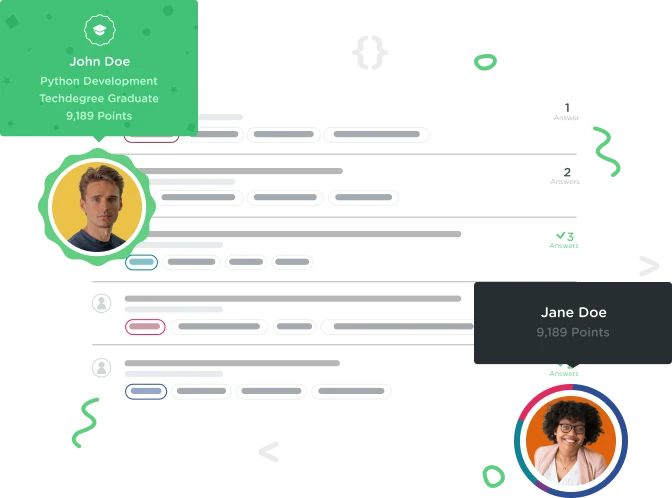

Adam Tyler
14,865 PointsWhat is the point of loc and iloc
In python pandas we can access values from a series as follows
import pandas as pd
balances = pd.Series( [20.00, 20.18, 1.05, 42.42], index=['pasan', 'treasure', 'ashley', 'craig'] )
balances.iloc[0] 20.0
balances[0] 20.0
Both will return the same thing. What is the difference.
Similarly,
balances.loc['pasan'] 20.0
balances['pasan'] 20.0
3 Answers
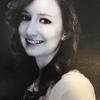
Megan Amendola
Treehouse Teacherloc gets rows (or columns) with particular labels from the index. iloc gets rows (or columns) at particular positions in the index (so it only takes integers).
Taken from this stackoverflow answer
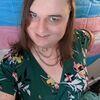
Renee Brinkman
Python Development Techdegree Graduate 13,572 PointsI believe what you're asking is "why use balances.loc['pasan']
when you can just use balances['pasan']
with less typing?" The way I understand it, if you don't use iloc
or loc
, whether you are specifying an integer or label as your index is implicitly determined by pandas, which could give you the wrong value, depending on what data you're working with.
Explicitly specifying iloc
or loc
, depending on how you're indexing the data, can ensure that you are getting the exact value you need.
Here is a code snippet that illustrates this:
import pandas as pd
data = pd.Series([30.2, 407.2, 23.0], index=['spam', 'eggs', 0])
print('Your data is:')
print(data)
print(f'Now notice that data[0] ({data[0]}) is not the same as data.iloc[0] ({data.iloc[0]})')

Gerald Bishop
Python Development Techdegree Graduate 16,897 PointsI think that loc and iloc are faster than not using them according to pandas documentation because they have less overhead in first determining the data type.
Taken from Pandas' docs:
While standard Python / NumPy expressions for selecting and setting are intuitive and come in handy for interactive work, for production code, we recommend the optimized pandas data access methods
Taken from Pandas' docs :
Since indexing with [] must handle a lot of cases (single-label access, slicing, boolean indexing, etc.), it has a bit of overhead in order to figure out what youβre asking for. If you only want to access a scalar value, the fastest way is to use the at and iat methods, which are implemented on all of the data structures.
Similarly to loc, at provides label based scalar lookups, while, iat provides integer based lookups analogously to iloc