Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial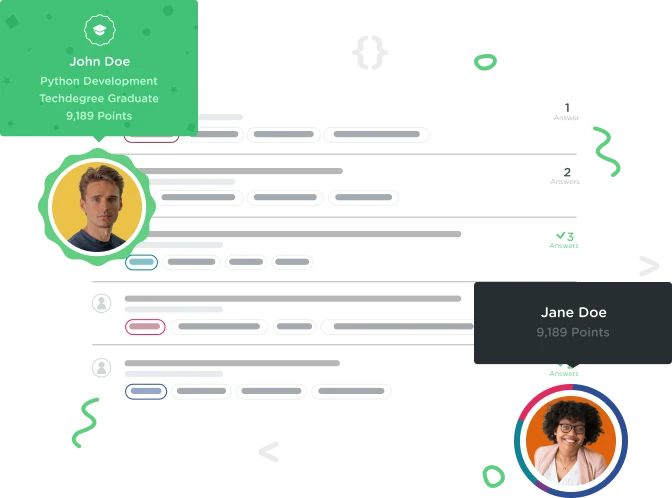

Ayo Ogunlana
457 PointsWhat is the point of Objects and Class in java??
I just want to get an in-depth understanding because Craig said this was the foundation to understanding Java.
5 Answers
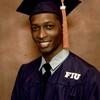
Dane Parchment
Treehouse Moderator 11,075 PointsHere hopefully this helps you better understand.
Objects: Objects are literally what you think they are, the programming counterpart of some real-world or abstract objects. Lets say that a car is an object. Well a car has a color, a set mpg, and a manufacturer; also a car can accelerate, decelerate, honk a horn, and turn. Well we can program this as well. We can create a "Car" Object that has a color variable, an acceleration variable, an accelerate method and a decelerate method, etc. etc. What you need to understand is that an object can have states and behaviors in our case the objects states are its: Color, MPG, and Manufacturer while its behaviors are: Accelerating, Decelerating, Turning and Honking!
Class: A class is basically a blueprint for an object, for example:
public class Car {
public int mpg;
public String manufacturer;
public String color;
//This method called a constructer will allow us to create an object of this class in another class (or even in this one)
public Car(int milesPerGallon, String company, String carColor) {
this.mpg = milerPerGallon;
this.manufacturer = company;
this.color = carColor;
}
public void turnCar() {
//Turns the car left or right
}
public void accelerate() {
//Accelerates the car
}
public void honk() {
//Honks the horn
}
public void decelerate(){
//Decelerates the car
}
}
From that example you can see how we created a class or Blueprint for a car. By using this class we can create car objects in our program. For example:
public class CarLauncher {
public static void main(String [] args) {
//Create a car object
Car toyota4Runner = new Car(22, "Toyota", "Black");
//Create another car object
Car camaro1969 = new Car(10, "Chevy", "Red");
//Make the cars accelerate and turn
toyota4Runner.accelerate();
toyota4Runner.turn();
camaro1969.accelerate();
camaro1969.turn();
}
}
As you can see we created two car objects using the car class as a blueprint!
Hopefully this helps you better understand If you need any more help understanding let me know in the comments!
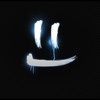
Grigorij Schleifer
10,365 PointsFantastic answer !
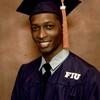
Dane Parchment
Treehouse Moderator 11,075 PointsThank You! :D

Ayo Ogunlana
457 PointsThanks, I understand it way better now.

Abbas Nowruzi
5,191 PointsThanks :)
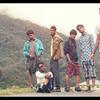
Sachu AA
526 PointsBrilliant Answer..Got the right explanation for Objects and Classes.. Thanks A lot . Dane Parchment.
Edit: Fixed my name ;) - Dane E. Parchment Jr. (Moderator)
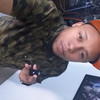
Bryan Martinez
414 PointsAmazing explanation! I was kind of lost but now I understand much better. THank you
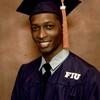
Dane Parchment
Treehouse Moderator 11,075 PointsGlad to have helped you out. If you are in need any other OOP concept explained, I can definitely do that.