Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial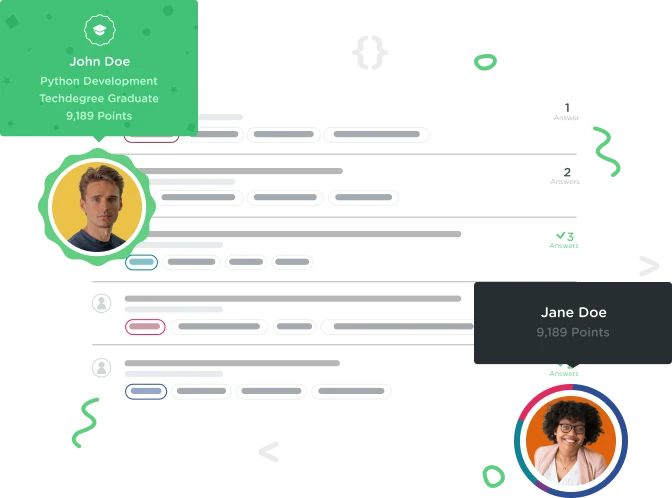
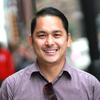
Jon Maldia
8,473 PointsWhat is the practical use of using Variable Functions?
Is there any practical use for using variable functions? I guess using it for testing functions but is there any other reason why you would want to create a variable function?
3 Answers
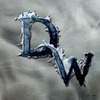
Hugo Paz
15,622 PointsYou could use it for a callback function.
Basically you pass a function to a function.
From the php manual
<?php
// Our closure
$double = function($a) {
return $a * 2;
};
// This is our range of numbers
$numbers = range(1, 5);
// Use the closure as a callback here to
// double the size of each element in our
// range
$new_numbers = array_map($double, $numbers);
print implode(' ', $new_numbers);
?>
The above example will output:
2 4 6 8 10
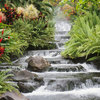
kabir k
Courses Plus Student 18,036 PointsAn important reason for creating variable functions is that they are very useful for callbacks.
And it helps to understand what variable functions are.
Using examples from the video, from the variable, $func, we can switch the function's name (as a string), from 'answer'
<?php
function answer( ){
return 42;
}
function add_up($a, $b){
return $a + $b;
}
$func = 'answer';
echo $func( ); // using echo to print the returned value of the answer function, 42 to the screen
to 'add_up'
<?php
function answer( ){
return 42;
}
function add_up($a, $b){
return $a + $b;
}
$func = 'add_up';
echo $func(5, 10); // using echo to print the returned value of the add_up function, 15 to the screen
The variable involved in a variable function is like a canister or container holding our function except that it holds the functionβs name as a string and can be switched out with another function to allow us to use the functionality of the new function i.e. the functionβs name it is currently holding (without having to have to modify the first function and thus we can have multiple custom functions that do different things)
That is, the string variables allow us to call pre-existing functions based on the functions' names as their values
And this concept makes the functions, variable functions.

William Twiner
2,605 PointsWhew still way over my head at this time of the course
Jon Maldia
8,473 PointsJon Maldia
8,473 Pointsexcellent! thanks so much hugo!
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 PointsHugo Paz Hi, can you give a easy example. This example crossed over my head.
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsHi @ammarkhan,
Maybe this example will help
So we create an anonymous function (closure) and assign it to the variable $double.
We then create an array with a few numbers.
We then create a function called doubleUp which takes as arguments a function ($func) and an array ($arr).
We then use them both to echo out the values on the screen.