Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial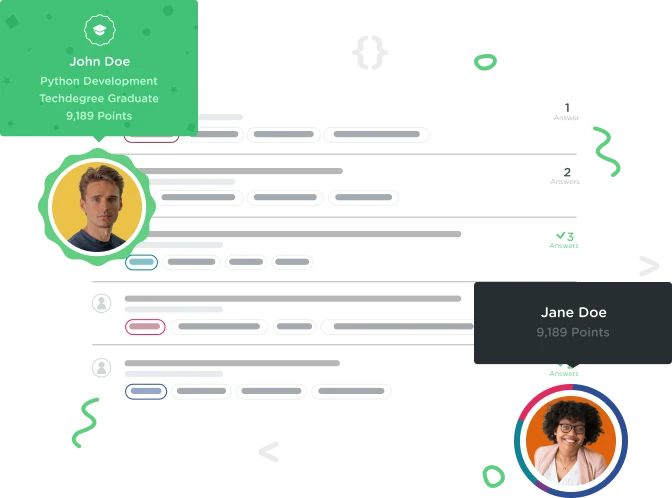

ido sh
3,926 Pointswhat is the problem?
OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you! Oh, be sure to look for both uppercase and lowercase vowels!
def disemvowel(word):
for i in word:
if i.lower() == 'a' or i.lower() =='e' or i.lower() =='i' or i.lower() == 'o' or i.lower() == 'u':
del word[i]
return word
1 Answer
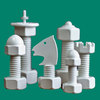
Steven Parker
229,744 PointsIn Python, strings are immutable.
So you can't modify them directly. But, you could perform operations on a string which generate a new string, and then re-assign the original string from the new one. Another idea might be to convert the string into a list, perform the operations on the list, and then convert it back.
I'll bet you can pick one of those approaches and solve it without a spoiler.
Grant Tribble
8,210 PointsGrant Tribble
8,210 PointsHi ido, It looks like you have a couple of hick-ups here. First, strings are immutable, so you'll have to change your input string to a list and then convert back to a string for the return. Next, we can simplify this process (even further than what I'll post for you) to clean it up and keep it straight forward. I've tried to follow the style you've got going with a couple of housekeeping adjustments. Always keep in mind what each type is and isn't allowed to do. Using the wrong data type can make almost any task into an impossible problem. I hope this helps.