Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial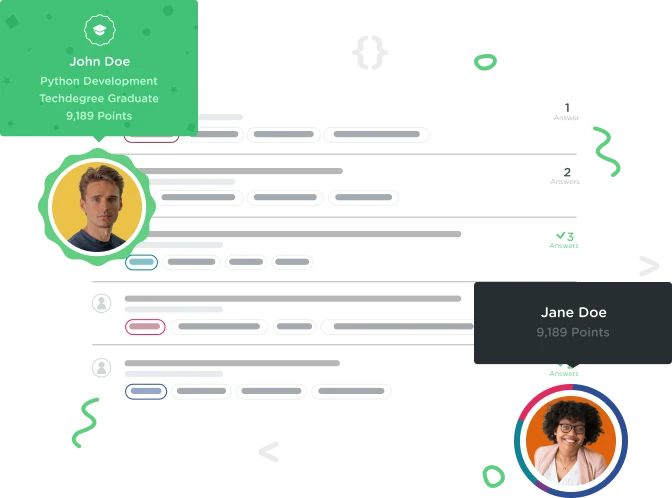

Miika Vuorio
3,579 PointsWhat is the problem with my reverse number game?
import random
def main():
guesses_left = 4
help = """If I guess too low write "higher".
If I guess too high write "lower".
If I'm correct write "correct"
"""
current_guess = random.randint(1, 10)
higher_or_lower = input("My guess is {} ".format(current_guess))
while True:
if higher_or_lower.lower() == "higher":
current_guess = random.randint(current_guess + 1, 10)
higher_or_lower = input("How about {} ".format(current_guess))
elif higher_or_lower.lower() == "lower":
current_guess = random.randint(1, current_guess - 1)
higher_or_lower = input("How about {} ".format(current_guess))
elif higher_or_lower.lower() == "correct":
again = input("Yay. Shall we go again Y/n: ")
if again.lower() != "n" or "no" or "no.":
main()
else:
break
else:
print(help)
higher_or_lower = input("Now tell me. Was I correct or should I guess lower or higher? ")
continue
guesses_left -= 1
if guesses_left == 0:
again = input("I'm out of guesses. Shall we go again Y/n: ")
if again.lower() != "n" or "no" or "no.":
main()
print("""Here's a fun game. Think of any integer between 1 and 10 and I'll try to guess it.
I have 4 guesses.
If I guess too low write "higher".
If I guess too high write "lower".
If I'm correct write "correct"
If you write anything that isn't a recognizable command I will show these messages again.
""".format(help))
play = input("Do you have a number and are you ready to play? Y/n ")
if play.lower() != "n" or "no" or "no.":
main()
else:
print("bye")
I have many problems with my code that I just can't locate. First of which being even if I type n or no in any of the parts where it asks shall we go again or do you want to play it just ignores it and plays again. Second being the higher and lower commands make no difference. It seems to create a random guess every time. And finally please tell me if I have any bad programming practices. Sorry if there was a lot to do in this post.

Miika Vuorio
3,579 PointsThere you go.
1 Answer

Luke Strama
6,928 PointsPerfect, that's a lot better!
I ran through the game a few times and I noticed a few things...
The reason your exit question is not working is because of the multiple 'or' statements you have. If you either eliminate the extras and have:
if play.lower() != "n" :
main()
.... your exit 'n' will work. The other way would be to add
if play.lower() != "n" and play.lower() != "no" and play.lower() != "no.":
main()
Also, the 'while True' in your main() should actually be 'while guesses > 0'. Then, if instead of having a 'when guesses_left==0', you create a 'Else' statement that is indented the same as your 'while guesses > 0' block. It'll run that block when guesses_left ==0.
Lastly, I would probably look to change how the computer comes up with guesses. A couple times, it ends up guessing the same number that it already guessed before. I think possible using sets and adding that to the logic in some way might help...
Luke Strama
6,928 PointsLuke Strama
6,928 PointsIf you could repost this with all of the code within the same script, it might be a little easier to see where this are. There's a few pieces that are just written in plain text and make it hard to figure out where in the code they belong...