Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial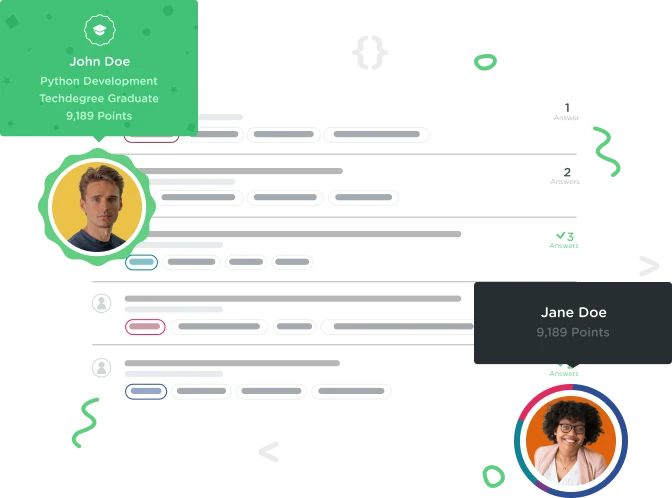
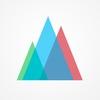
Chris Mason
Full Stack JavaScript Techdegree Graduate 24,526 PointsWhat is the purpose of certain words between parenthesis
This code works in the browser, but I'm struggling to understand where (message) and (songs) fit into this. For example, I don't see how (message) in the print function relates to anything that's going on in the code. I know it is important, as when I comment it out the code doesn't work, I've just a gap in my knowledge which I hope someone can help to fill! Many thanks :)
var playList = [ ['I Did It My Way', 'Frank Sinatra'], ['Respect', 'Aretha Franklin'], ['Imagine', 'John Lennon'], ['Born to Run', 'Bruce Springsteen'], ['Louie Louie', 'The Kingsmen'], ['Maybellene', 'Chuck Berry'] ];
function print(message) { document.write(message); }
function printSongs( songs ) { var listHTML = '<ol>'; for ( var i = 0; i < songs.length; i += 1) { listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] +'</li>'; } listHTML += '</ol>'; print(listHTML); }
printSongs(playList);
4 Answers

rakan omar
15,066 PointsBenjamin does a really good job explaining the code, but considering your question we can see that you don't yet understand what arguments are, so I'll just explain that.
when you have a function and there is words between its parenthesis, these words are kind of like declaration of local variables that are used inside the function. when you call the function, you give these arguments - which are like variables - their values. Let me give you a reallyyy simple example to help you understand arguments
function addNumbers(firstNumber, secondNumber){
return firstNumber + secondNumber;
}
var sum = addNumbers(2, 3);
when this code runs, the variable "sum" will have the value of 5. because "firstNumber" was passed the value 2, and secondNumber was passed the value 3, and the function then added the two arguments together, returning 2+3 which is 5. so if you called it like this addNumbers(200, 500); it would return 700

Benjamin Barslev Nielsen
18,958 PointsBelow I have explained how the code works:
Let's start by looking at the print function:
function print(message) { document.write(message); }
print takes a string message as an argument and calls document.write(message). document.write writes a string to the browser, i.e., it is that call that shows the playlist in the browser. Therefore it doesn't work if you comment it out.
Now let's look at the printSongs function:
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] +'</li>';
}
listHTML += '</ol>';
print(listHTML); }
This function takes an array songs, which it uses to create an HTML string, so line 1-5 inside the function just creates the HTML which represent the songs. Since we now have the HTML string containing information about the songs, we can call the print method (line 6 inside the function), which then displays the songs list in the browser. This is what happens since message in the print function now contains the HTML string saved in listHTML.
Now all we need is to call the function printSongs with the playList we would have shown in the browser:
printSongs(playList);

Michael Moffet
7,646 PointsJust one point to add on top of the previous responses that a professor of mine used to really stress is that the names of the arguments passed in do not matter, only the order. Let me explain with an example:
if you have this function from the above answer:
function addNumbers(firstNumber, secondNumber){
return firstNumber + secondNumber;
}
those two argument names only exist within the function. So when you call it, you can pass in whatever variables you want and it will assign them to those arguments in the same order. So if you have two variables like this:
var num1 = 5;
var num2 = 10;
You could call the function like so:
addNumbers(num1, num2);
And when the function runs, it's going to assign those variables to the arguments in the same order they're listed in the function. So firstNumber becomes num1, secondNumber becomes num2.
And if you reverse the order when you pass them in like this:
addNumbers(num2, num1);
It's still going to assign them in the order they're listed. So now firstNumber is num2, and secondNumber is num1.
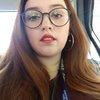
Camila N
10,677 Pointsall the answers above are really good! I just came to recommend the JavaScript Basics course. It explains a lot of things related to functions. Given that you didn't know what arguments are, perhaps there are still gaps in your knowledge about functions and you could benefit of watching the last section of the course I mentioned before continuing with this one. Good luck!