Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial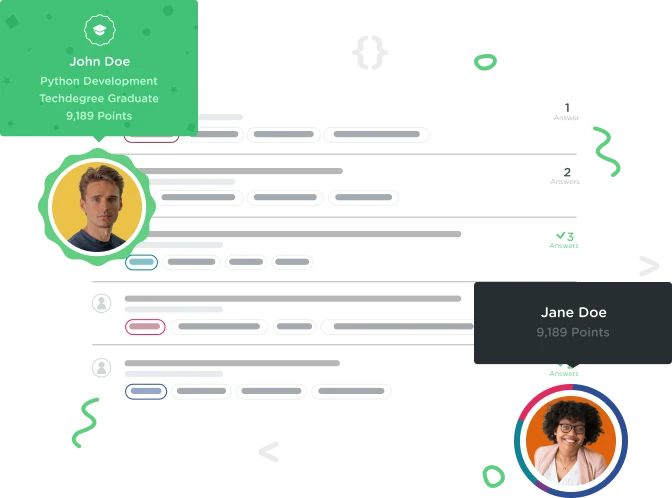

Yan Kozlovskiy
30,427 PointsWhat is the purpose of initializers and the self keyword?
I don't understand the point of creating an initializer. Why would we need to do this when Swift handles this automatically. Also, what is the purpose of the self keyword and how does it work? I watched the video a few times over and I still don't get it. The only thing I got from it is that it refers to the current instance, but I don't really understand why or when to use it.
2 Answers

Wing Sun Cheung
4,933 PointsGreat question,
First of all, I completely agree with you, I mean why the heck would we need an initializer and whats up with the self keyword?! It is all very confusing.
Well, think of it this way. A class splits into states (properties) and behaviors (methods), and at the point of the object creation, the states must be defined. So, how to do make sure they are define? well, we could define the property right at the beginning, for example:
class UserProfile {
let firstName: String = "Steve"
let lastName: String = "Jobs"
}
so when we instantiate the UserProfile
object, say let steveJob = UserProfile()
, it is ready to use. You might be asking, why the heck you would want to set a default name in a class like the UserProfile
, correct, you wouldn't! because not all users are Steve Jobs. So, we do this:
class UserProfile {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
}
Hey, it is that ugly init
thing again! The init method allows us to input what the firstName
and lastName
should be at the point of instantiation. If we don't have that init
, how would Xcode know what to put in there? remember, when we instantiate an object, that object must be fully functional, meaning all the states (properties) which in this case are the firstName
and lastName
must be fully define.
Now, the self
keyword refers to the object itself, the reason we need to put self is because since the init
method's parameter name is the same as the property name, we need to be clear that the property we are assigning values to is from this class itself. So. the literal translation of self.firstName = firstName
would be:
"Hey Xcode, please use my own (self
) stored property firstName
and assign the value firstName
to it, I know they are the same name, that is why I am using the keyword self.
Hope this makes sense :) Happy coding,
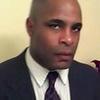
Ingo Ngoyama
4,882 Pointsinitializers are a way for you to customize how the class you write is called by objects by using the variables that you choose to be the go between of the object and the class to hold the values that you have set as soft code . it allows you also to set values.
init( car: String, speed: Int = 30)
{
self.car = car
self.speed = speed
{
1)What is happening here is that the self.car is the car variable that is in the class.
2)The car being initialized in the parentheses is making a variable for an object to use as call to the car value in the class and setting the type as String.
3) We set it so that what ever value that is put in the object for car ( car) will be assigned to the car in the class (self.car) and then it will work whatever algorithms we have with that value and then object can get the return result.
4) further more you can also use inits to set default values like a cruising speed for the car of 30mph so when the object first calls it automatically is at a base speed and not zero.
In short inits are like like adjusting frequencies on both ends of walkie-talkies so you can communicate to each other how they will begin some operation.