Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial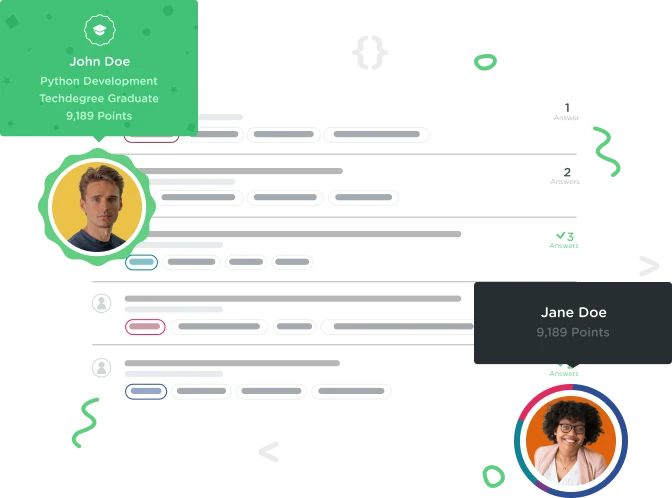
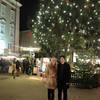
nicholas maddren
12,793 PointsWhat is the question asking of me?
I feel as if I've just watched a different video for the actual task.
Here is my code:
function arrayCounter(array){if
(typeof === 'Undefined')
return 0;
}
return array.length
};
And here is the question:
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
I haven't learnt about adding a parameter which is an array yet so I don't know how to do this? Any help would be great :)
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsPassing an array to a function is in no way different from passing a variable with any other data type.
Let's say you have an arrayPrinter
function that prints an array. It doesn't matter how it works or even what it does. It takes a single parameter — an array. You can pass that parameter as a variable or as an array literal:
var myArr = [0, 1, 2, 3, 4, 5];
arrayPrinter(myArr); // just like any other variable
// or
arrayPrinter([0, 1, 2, 3, 4, 5]); // an array literal is still a single parameter
Now, as the task says, you have to check if the parameter passed to the function is a number, string or undefined. If it is one of those, you return 0, otherwise you return the length.
Your first attempt is actually somewhat close to what you need to do. It's important to know that the typeof
operator needs to work on something. To check if something is undefined, you need to specify that something:
if (typeof paramName === "undefined") {
// do something
}
Also, typeof
returns all lower-caps strings.
Next, you need to use the logical or operator (||
) to do all of those checks in a single if. You can read about how that works in another thread.
So, when you combine all of that, you get something like this:
function arrayCounter(array) {
if (typeof array === "undefined" || typeof array === "number" || typeof array === "string") {
return 0;
}
return array.length;
}
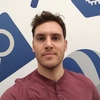
Philip Cox
14,818 PointsMaybe something like this?
functionarrayCounter(array) {
if ( Array.isArray(array) ) {
return array.length;
} else {
return 0;
}
}
Philip Cox
14,818 PointsPhilip Cox
14,818 PointsSorry to hijack the thread, but how do you add those code snippets please?
Dino Paškvan
Courses Plus Student 44,108 PointsDino Paškvan
Courses Plus Student 44,108 PointsCheck the Markdown Cheatsheet for more info, but the gist of it is that you need to use three backticks (```), not three single quotes.
Jon Edwards
7,913 PointsJon Edwards
7,913 PointsCan anyone please explain why sometimes you use the "else" in the function, while other times it seems that it is just implied and the else is not even typed in? Is it simply a shorthand version? I've seen many solutions to this problem on the forums, some using else, others just leaving it off. Thanks!