Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial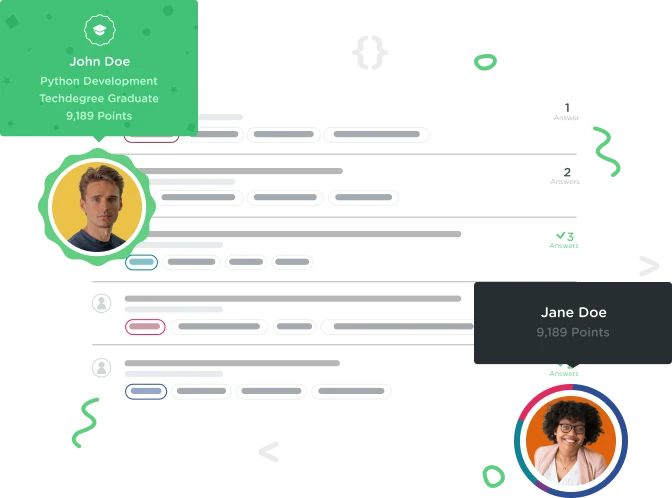

Anika Mehta
107 PointsWhat is the reason behind creating constructor? Please help
What is the reason behind creating constructor? Please help
3 Answers

Yanuar Prakoso
15,196 PointsHi Anika
The fastest answer I can give you is constructor exist to enable the class to instantiate (create) object whenever it is called and set the object created with attributes. The class give the blueprint of how the object will be like and the constructor created it. Constructor is often used to fill the variables declared in the class (usually private variables) using either arguments given by user or set inside the constructor it self. So if your class is not required to create an object out of it, for example the class which has method called Main in it, it does not need a constructor. If you already satisfied with the explanation you can skip the rest because it is kinda long:
Since you have reach the Hangman project I suppose you have finish your PezDispenser project. Let us use that to show what constructor do. Mind you that this is just simple small example of what constructor do okay. i am still learning also here so bear with me:
class PezDispenser {
public static final int MAX_PEZ = 12;
final private String characterName;
private int pezCount;
public PezDispenser(String characterName) {//<--this is constructor
this.characterName = characterName;
pezCount = 0;
}
In the example above both PezDispenser class and method share the same name but the method accept arguments in form a String called characterName. This is the requirement to be a constructor method. Above the constructor you can see that there are variables declared and most of them private meaning outside method cannot directly access it. By putting this.characterName = characterName the constructor class set the value of variable characterName using an argument from outside the class. Meanwhile the second private cariable pezCount is set directly within the class to be equal to zero.
Meaning each time any class outside PezDispenser class calls it using: (I use Example.java from Pez Dispenser project)
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new PEZ Dispenser");
System.out.printf("FUN FACT: There are %d PEZ allowed in each dispenser %n", PezDispenser.MAX_PEZ);
PezDispenser dispenser = new PezDispenser("Yoda");//<--this is calling the constructor of PezDispenser to set an object called dispenser which is a PezDispenser with "Yoda" character as its head
Here in the Example class you call the PezDispenser constructor to make an object called dispenser which has features as PezDispenser. However, there is one feature that Example class set that is the characterName which in this case "Yoda". Thus by calling the constructor method and pass the argument "Yoda" you have created a new object with features as presented in PezDispenser class. That is the main function of constructor method: construct what the object the class will be created. The class give the blueprint of how the object will be like and the constructor created it.
As you can see since Example class does not required to be used as constructor of any object it does not have constructor method. It has only public static void main method which the compiler will set as the beginning of where the code will be executed.
I hope this will help a little.

Yanuar Prakoso
15,196 PointsHi Mehta...
You should see it from another angle I guess. PezDispenser class is the blue print of object called dispenser. Anything that we can do with dispenser and how dispenser is set is set in the PezDispenser class. Thus you will never see dispenser to be used inside the PezDispenser class. About how you passed the "Yoda" nameCharacter it was passed when you wrote this code : new PezDispenser("Yoda"). Then the compiler will search for PezDispenser class and its constructor. The constructor is written in code as public PezDispenser (String characterName) right? The code written in the bracket will let the Java compiler knows it expect the Example class which created the dispenser object need and will pass "Yoda" character name.
Then the constructor pass that "Yoda" characterName to be attached inside the dispenser object. Therfore, although you never see dispenser inside the PezDispenser class the dispenser is already created with special feature which is "Yoda" as character name.
The other way to describe it is to look PezDispenser class as factory that make Pez Dispenser. The PezDispenser factory lets you as customer to add your own character name as special feature to be attached as the head of the Pez Dispenser.
Then you as customer order the customized Pez dispenser through online store called Example class. The Example class then designate your order as new unique name dispenser. Then the Example store asks you what charachter name you want as the head of the dispenser (your special new Pez Dispenser). You answer "Yoda" and then Example class online store pass your request to PezDispenser class factory. PezDispenser class factory make the dispenser exactly as your specific request with "Yoda" character name and send it back to Example class online store to be given to you as customer. After this the job of PezDispenser class as factory ends and you have your new Pez Dispenser called dispenser. You can fill it with candies. Eat candies from it etc. It is all specified inside the PezDispenser class as blue print.
I hope this will help a little. It is rather difficult to grasp Java terms and concepts in early stage of learning. However, as you code and code more your body will gain understandings on how it is doing. It is just like learning a language to speak. You might not fully remember what is the logic behind the language rules but your brain remembers the patterns on how to speak the language.

Anika Mehta
107 PointsThank you so much. I am trying really hard to learn from core java. It is taking a lot time

Yanuar Prakoso
15,196 PointsThat is okay. Take your time do not hesitate to explore and the most important is to keep coding. At least in my case the longer my fingers type codes the better understanding of the pattern on how to program I get. As I said it just like learning language, you need to speak the language not just listening other people explaining the language.
Happy coding
Anika Mehta
107 PointsAnika Mehta
107 PointsPezDispenser dispenser = new PezDispenser("Yoda");
As this creates object dispenser, we never used this object in PezDispenser class then how does "Yoda" is passed as value? What is the use of creating this object as we never used it?
<noob />
17,062 Points<noob />
17,062 PointsAmazing answer, thanks for this explantion, u have made alot of things clear to me :D