Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial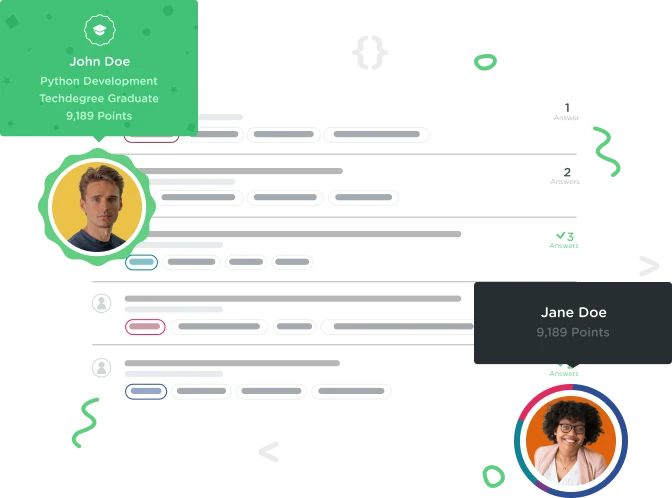

Ralph Wong
2,103 PointsWhat is the reason for self.config?
In the video, Pasan says that because the var config is being loaded using the "lazy" fashion, you need to put self in front of config when calling the variable in the NSURLSession method. This would suggest that by taking the " lazy" away when creating the var config, you would no longer need the "self". However, this is not the case as I get error messages when I do that. If I take lazy off when creating the session variable and the "self" off, I still get an error.
This code will generate an error that NetworkOperation.Type does not have a member named 'config':
var session: NSURLSession = NSURLSession(configuration: config)
So I don't understand why putting "lazy" in front when creating the session variable and a "self" in front of the config works, but taking away both the "lazy" and "self" does not work. Can anyone help?
1 Answer
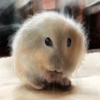
Dominic Pettifer
4,338 PointsI'll attempt to explain this based on my understanding of how Swift works from a book I've read, but someone correct me if I'm wrong.
You need self.config because Swift can't determine if you're referring to an instance stored property (stored against an instance of NetworkOperation) or a class/static property. I'm not sure static properties have been covered yet in this course, but essentially they are stored against the class itself, instead of instances, and all instances will get the same value. You use static properties like so...
class NetworkOperation {
static var thing: String = "Hello world!"
}
let thing = NetworkOperation.thing
Note that we don't have to create an instance of NetworkOperation to access our 'thing' property.
If you wanted to omit self. you could write your code like so...
class NetworkOperation {
static var config: NSURLSessionConfiguration = NSURLSessionConfiguration.defaultSessionConfiguration()
var session: NSURLSession = NSURLSession(configuration: config)
}
This will compile and run fine, but be warned, all instances of NetworkOperation will get the same NSURLSessionConfiguration instance.