Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial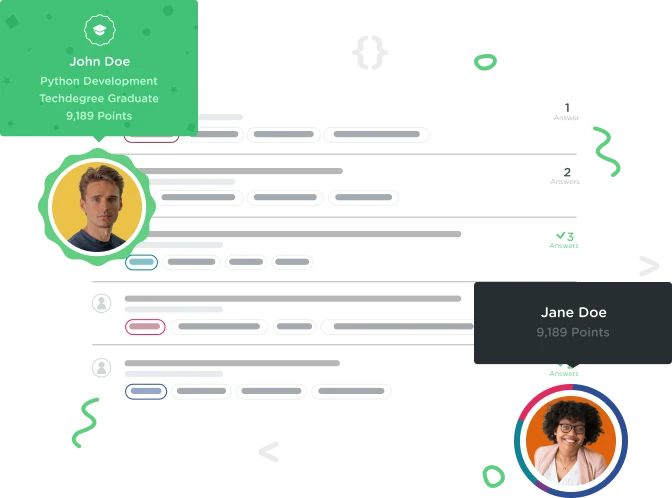
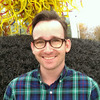
Max Pedersen
7,196 PointsWhat is the reason for setting var randNum?
First off, I'm loving Dave's classes. They are very thorough, and the examples/quizzes/challenges are relevant and help reinforce the information.
In the while loop presented in this video, Dave creates a variable called randNum that simply holds the result of the randomNumber function:
while (counter < 10) { var randNum = randomNumber(6); document.write(randNum + ' '); counter++; }
Would it not be cleaner/simpler to use the following?
while (counter < 10) { document.write(randomNumber(6) + ' '); counter++; }
I'm new to JavaScript--was Dave just spelling things out a bit more because this is a beginner class, or is there a reason for doing things this way rather than the way I did it?
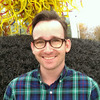
Max Pedersen
7,196 PointsThanks, Jessica! Very helpful, and now that I'm a bit further into the course, I think we're right--it looks like he was just simplifying things for clarity's sake. Cheers!
1 Answer
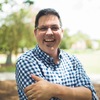
Dave McFarland
Treehouse TeacherHi Max Pedersen
Jessica Barnett is correct (good answer, by the way, Jessica). You can often write shorter, and more compact JavaScript code, but I find throwing too many things into one line of code can confuse people (even advanced programmers).
For example, this line of code opens a prompt, asks for a name, converts it to uppercase, combines it will another string and prints it out to the document window:
document.write("Hello" + prompt("what is your name").toUpperCase());
But that's a lot to take in. And, I find something like this clearer to understand:
var userName = prompt("what is your name");
var message = "Hello " + userName.toUpperCase();
document.write(message);
Of course you could write this in other ways:
var userName = prompt("what is your name").toUpperCase();
document.write("Hello " + userName);
The most important thing for your programming is making sure that you can understand what your code does. You should also think about the future when you come back to your code. Readable code is better code. Shaving off a few lines of JavaScript code is not going to give your program a performance boost.
Jessica Barnett
8,028 PointsJessica Barnett
8,028 PointsI could be wrong, but I think it was probably just him simplifying things so they're easier for people to understand, like you said.
You could also do this with a for loop, which would be my go-to if I had to pick the best way.
Also worth noting - I've heard that it's generally not the best idea to create a variable inside a loop like in the example. Better to define it once, outside, so that you're not redefining a new variable 10 times.
It works faster, though computers are so fast these days that the difference is pretty negligible, especially for something like this. Just thought you might like to know.
Love that you're picking at the details in trying to understand this. I tend to approach things the same way, and I think you get a much more thorough understanding that way. Hope it works out for you too. Good luck!