Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial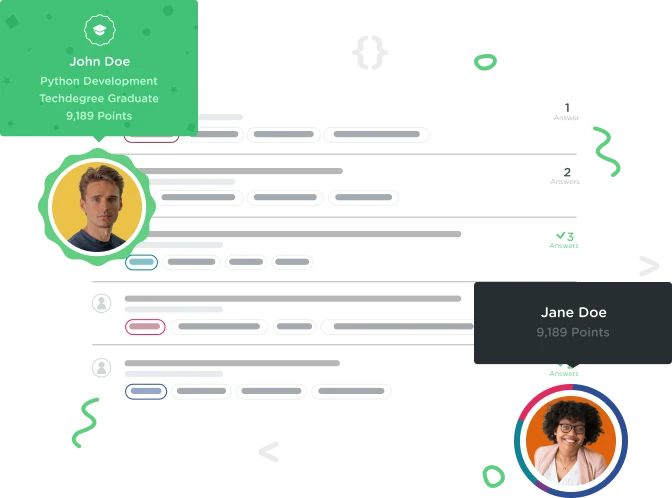

Simon Kassa
3,558 PointsWhat is the recommended/standard way of identifying arrays from other type of objects?
I came across a post on stackoverflow on the topic of checking if an object is an array. Some of the discussion posts were specific to arrays and others were general object identification. I want to know if there is a recommended way of identifying arrays or other objects?
Link: http://stackoverflow.com/questions/4775722/check-if-object-is-array
5 Answers
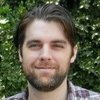
Michael Liquori
8,131 PointsAccording to MDN it seems to be:
Array.isArray(my_array) === true/false
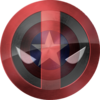
J Scott Erickson
11,883 Pointstypeof will give you a returned string to compare against for simple data types (strings, integers). Array is different. This works for example:
var arr = ["this","and","that"];
if ( arr instanceof Array ) {
console.log("Yep, thats an array");
} else {
console.log("Nope, thats not an array");
}
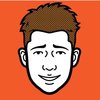
Arthur Verschaeve
20,816 PointsI'm not a huge fan of using the instanceof
operator for this, since it doesn't work with Array-Like Objects. Also, since array isn't a datatype something with typeof
won't work.
I personally prefer using something like this function (grabbed from the jQuery source code)
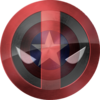
J Scott Erickson
11,883 PointsI'd agree that this is the better way if you are using the jquery library in your project.
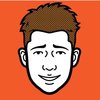
Arthur Verschaeve
20,816 PointsYou don't have to be using jQuery for that, it's like one function that you can just copy.

Faddah Wolf
12,811 Pointsi'm with Michael Liquori above ^^^^. per MDN it's Array.isArray().
ā faddah portland, oregon, u.s.a.

diannalord
7,284 Pointslonger version
function isArray(myArray) {
return myArray.constructor.toString().indexOf("Array") > -1;
}