Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial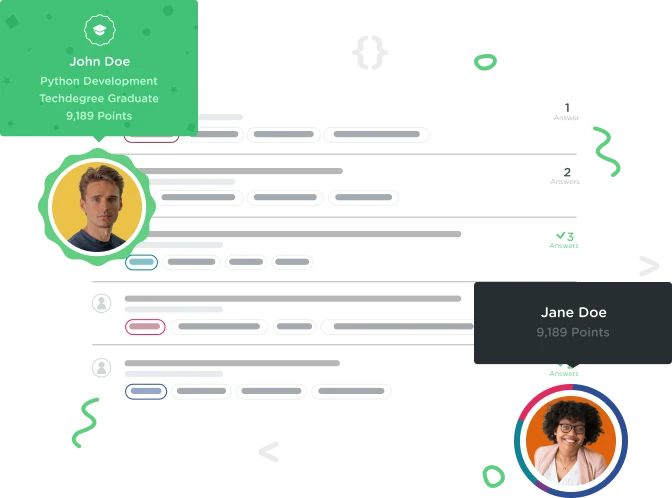

Daesik Kim
5,422 PointsWhat is the 'right datetime'? Please tell me what is wrong with this code.
def time_tango(date, time): now = datetime.datetime.now() date = now.strftime('%Y-%m-%d') time = now.strftime('%H:%M:%S:%f') dt_str = date + " " + time dt = datetime.datetime.strptime(dt_str, '%Y-%m-%d %H:%M:%S:%f') return dt
How should I fix this?
import datetime
def time_tango(date, time):
now = datetime.datetime.now()
date = now.strftime('%Y-%m-%d')
time = now.strftime('%H:%M:%S:%f')
dt_str = date + " " + time
dt = datetime.datetime.strptime(dt_str, '%Y-%m-%d %H:%M:%S:%f')
return dt
3 Answers
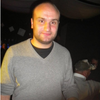
Vittorio Somaschini
33,371 PointsHello Daesik.
What I meant is that you need to get rid of that now variable and everything related to that.
The main idea you have is good I think as you first wanted to get a date string and then a time string and in the end putting them together in the right form as requested by the code challenge.
Let see the case of date: You produced this piece of code:
date = now.strftime('%Y-%m-%d')
So, what I suggest is just get rid of this now variable that you have created as I do think we don't need it. We can still use the date variable, but we need to assign it based on the date value that the function takes, the code would then be:
date = date.strftime('%Y-%m-%d')
This will provide us with a string based on the date.
Same thing will apply to the time variable you want to create.
The rest of the code, where you put together the two instances, looks fine.
Please note that this is probably NOT the most elegant way to handle this as Ken suggested, but I thought your code was close enough to correct it a little and have it pass the challenge, as it is really close.
Vittorio

Ken Alger
Treehouse TeacherDaesik;
I would take a look in the Python documentation for the implementation of the combine method for datetime objects. That might get you headed in the correct direction.
Post back if you are still stuck.
Happy coding,
Ken

Daesik Kim
5,422 PointsI am not sure what kind of output I should get. This is what I did based on your comment.
import datetime
def time_tango(date, time): d = datetime.date(2015, 4, 1) t = datetime.time(18, 50) dt = datetime.datetime.combine(d, t) return dt
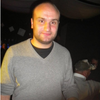
Vittorio Somaschini
33,371 PointsHello Daesik.
You are actually pretty close, I only see a concept mistake, but your code is close to the solution.
Here is the problem.
You are setting a variable called "now" which stores the time at moment "now" which is not needed at all. We only need to consider date and time in this challenge as they function will take those as parameters. You only need to get rid of the now variable code line and then set date and time like you did with the strftime method BUT based on the date and time taken by the function and NOT on the now variable.
I hope I made it clear.
Vittorio

Daesik Kim
5,422 PointsIs this what you meant?
import datetime
def time_tango(date, time): d = datetime.date(date) t = datetime.time(time) dt = datetime.datetime.combine(d, t) return dt
Daesik Kim
5,422 PointsDaesik Kim
5,422 PointsThank you very much! Finally solved!!!!