Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial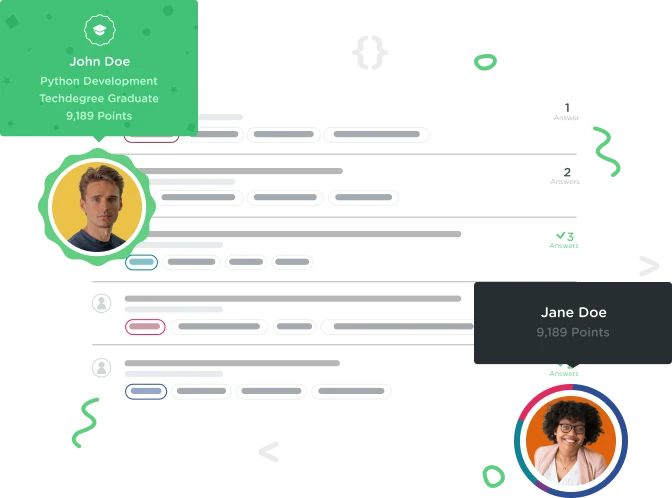
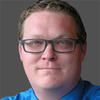
Jade Hudson
6,859 PointsWhat is this? In normal speak.
I know that this exercise is done correctly, but what is happening here?
Can anybody explain this in normal language?
The example of a pez dispenser or goKart battery is mucking it up.
What does this actually mean? Or what is happening in this code?
public class GoKart {
private String mColor;
public static final int MAX_BARS= 8;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
}
public String getColor() {
return mColor;
}
public void charge (){
mBarsCount = MAX_BARS;
}
}
5 Answers

Daniel Hartin
18,106 PointsHi Jade
OOP (object orientated programming) is tricky to grasp but very powerful once you understand the principles. I'll try to explain what is happening although truth be told explaning stuff has never been by strongest suit :) (you can always message back and i'll try to reply)
Okay so the entire block of code you have posted is a CLASS. A class is the way in which we group variables and methods together to manage data easier by creating OBJECTS. Objects and Classes is often where most people get muddled up; a class is the blueprint or instructions on how an object should work and what data an object should hold. The example I like to use is LEGO (everyone knows what lego is). A single lego brick is an object it has properties (this would be variables in programming jargon) like number of bumps, shape and colour. The mould used to make this lego piece would be the class in this example it doesn't do anything other than create objects.
Hopefully you're with me so far....
public class GoKart {
private String mColor;
public static final int MAX_BARS= 8;
private int mBarsCount;
}
The code block above shows you the variables of the class, now to avoid baffling you further ignore the MAX_BARS variable as this is what we call a static constant and only confuses what I'm going to explain.
Now the class or blueprint knows how to make go karts it knows they should have a colour and a battery life percentage but it doesn't know what these will be... (if you think about it how would the factory ever know before hand how much battery life will be in a gokart and what colour it will be until the gokart is made).
So how will the class ever know which object to make? We provide ways for us to communicate to the blueprint and alter parameters in order to make a GoKart which matches our needs at that time. These are the methods....
public class GoKart {
public GoKart(String color) { //Constructor - This is called when we create a new GoKart OBJECT from this class
mColor = color;
}
public String getColor() { //This is a method that this class gives to all objects just like the variables
return mColor;
}
public void charge (){ //This is the same as getColor()
mBarsCount = MAX_BARS;
}
}
Here there are 2 types of methods the one labelled as a constructor is a method we call when we create a new object from this class so at runtime we might want a 'blue' GoKart so we would create something like
GoKart myNewGoKart = new GoKart("blue");
We are saying here "I want a new GoKart object called myNewGoKart. I want it to be blue" the code goes to the GoKart class and says "hey, how do I make a GoKart? ... The GoKart class creates a new GoKart OBJECT by copying itself in effect and running the code inside the constructor (in this example being passed in the parameter blue) ... The class then comes back and says Here you go, a brand new GoKart in blue as you requested".
The second type of methods are simply methods that the class copies to the new object usually for the object to have the ability to manipulate it's own variables in order to interact with your code as you could place it anywhere.
I really hope I've explained some principles okay for you to understand but please message back if something doesn't make sense. The major benefit of splitting code up like this is it allows you to deploy these objects in numerous places within your code while only editing the code in one place so should a bug be in your code you know exactly where to look, it's confusing at first but very powerful and logical once it 'snaps' into place.
Daniel
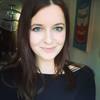
Marina Alenskaja
9,320 PointsAh okay, I'll try to explain :-)
public GoKart(String color) {
mColor = color;
}
This method takes whatever color we put in as an argument (color) when we call the method, and then it assigns it to the mColor variable. So if you picture a little gokart toy in front of you that is transparent - and you want it to be red - then you pass in red and the method takes the color and stores it in the color member of the object (mColor).
"Is that why this object is a better idea than individual code?"
An object is used to model something. It's extremly usefull, since you can't just write a buch of code and make it all "work together". An object can be thought of like a puzzle - all the pieces are code that models the picture. When it all comes together, you see a finished picture.
"The method that returns nothing" - I'm assuming you are referring to this:
public void charge (){
mBarsCount = MAX_BARS;
}
This method is created to perform a function in the background without giving us anything back. In theory, you could make it return a string after the battery is charged, for example "Battery fully charged" and just switch out the "void" with "String".
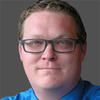
Jade Hudson
6,859 PointsThinking about the goKart as clear really helps here.
And am I right thinking that charge is simply stopping the charge process, or removing that code with void, once mBarsCount is at maximum?
The fact that charge wasn't mentioned or utilized in another part of the object makes me especially confused on that one.
I would understand a string that reported "fully charged", but it this one just keeping track of charge and storing this info for future use?
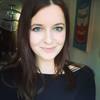
Marina Alenskaja
9,320 PointsHi Jade
Okay let's to try look at the code line by line.
public class GoKart { /*This is our gokart object. In here, we put everything related to the gokart,
like it's methods (what it can do) and variables (which properties we can play with).*/
private String mColor; //Here, we declare a private variable of the type string, that defines the gokart color.
public static final int MAX_BARS= 8; /*This is a private variable of type int (numbers) - it tells us
how much battery the gokart can have at maximum capacity (just like the bars on our phones).*/
private int mBarsCount; //This variable is how many bars there are currently (in the battery).
public GoKart(String color) { //Here, we define a method that takes one argument: a color. So if we pass in red - the gokart will turn red!
mColor = color;
}
public String getColor() { //This method simply gets the color of the gokart, in case we don't know what it's set to.
return mColor;
}
public void charge (){ //And finally, this method returns nothing - it just charges the battery to max capacity :-)
mBarsCount = MAX_BARS;
}
}
I hope this helps :-)!
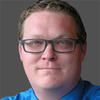
Jade Hudson
6,859 PointsHey Marina,
I understand those things now that you explained them, but what is the overall purpose of some of these things?
For example, why would I do this?
public GoKart(String color) { mColor = color;
Is this referencing a readLine input prompt on another page?
And then is it feeding it into the object to return a value?
Is that why this object is a better idea than individual code?
What is the overall purpose of an object like this?
And why would I create a method to return nothing, like this:
public GoKart(String color) { //Here, we define a method that takes one argument: a color. So if we pass in red - the gokart will turn red! mColor = color;
That's unclear.
I know what methods and variables do, but what is the process behind this code? That's more of what I'm asking.
--Jade

Tom Lawrence
8,685 Pointspublic GoKart(String color) is not a method really, its a constructor. (note how it has no return, and same name as the class). Maybe be better to call them constructors rather than methods so people do not try to add return types to constructors, or leave them off from methods.
Plus you cant call it like a normal method.
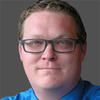
Jade Hudson
6,859 PointsThank you for your help.
I'll sit down with this later and try to understand.

Tom Lawrence
8,685 PointsThis is a constructor, every class has a default constructor which is not used in your example, but looks like this:
GoKart myKart = new GoKart();
You do not need to use the default constructor though, as sometimes it makes sense to do some custom stuff when you create a new object, like for example set its color which is exactly what your one is doing here (NOTE, previous posts state this is a methof. THIS IS NOT A METHOD, it is very different. you can tell it apart as it has the same name as the class, and no return type:
public GoKart(String color) {
mColor = color;
you would your constructor a bit like the default one above, but passing in a String like so:
GoKart myKart = new GoKart("Red");
Whatever String you pass in is then assigned to the the property mColor because of this statement in the constructor:
mColor = color;
The reason you would do this is because it makes creating a GoKart, and setting its color nice and easy.
Also, its worth noting that there is a rule where we should never directly modify a classes property directly, this is all part of something called encapsulation. The idea is, if you dont access something directly, you cant accidently change something.
This constructor does this, as your not directly assigning a color to mColor , the constructor is!
If you ever want to find out what the color is, again you wouldnt work with mColor directly, you would use something called a getter, this is simply a method which does the "getting" for you. Thats what this does:
public String getColor() {
return mColor;
}
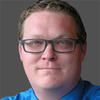
Jade Hudson
6,859 PointsAh. So that's the difference.
I was wondering how a constructor differed.
Thanks for your help!
Jade Hudson
6,859 PointsJade Hudson
6,859 PointsThanks Daniel. I'll give this a good read when I'm off work.
You're right that this is kind of difficult to process at first.
Jade Hudson
6,859 PointsJade Hudson
6,859 PointsThanks for the detailed explanation Daniel. This is really cool.
BTW, I think you're great at explaining it. I just think Craig's lesson obscures a little bit with its pez dispenser example. I know how those work, but thanks to you I have a bit more insight into what I'm doing with Java.
You rock my man.