Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial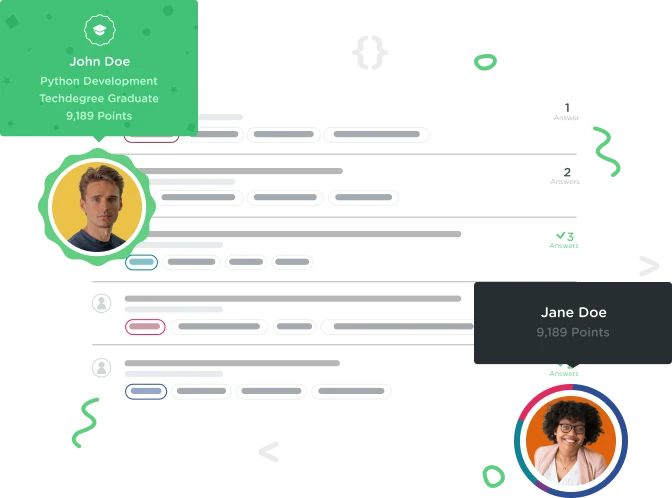

Anthony Walker
4,448 PointsWhat is this question on tuples looking for?
Not got a clue how to answer this question. Tried whole combination of things to no avail
4 Answers
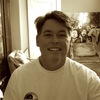
Anthony Price
1,412 PointsStone/Anthony -
How did you get task 2 to work? It won't accept it for me. Stuck. Thanks in advance!
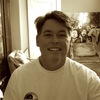
Anthony Price
1,412 PointsHere is the answer:
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting("Tom")
println(result.language)

Steven Prescott
19,206 PointsIf anyone gets stuck on this, make sure that you have
-> (greeting: String, language: String)
```
instead of reversing the two and putting (wrong)
```swift
-> (language: String, greeting: String)
```
It's an annoying mistype that took me a minute to catch.

Stone Preston
42,016 Pointstask 1 states: Currently our greeting function only returns a single value. Modify it to return both the greeting and the language as a tuple. Make sure to name each item in the tuple: greeting and language. We will print them out in the next task.
so instead of returning a string you need to return a tuple. thats an easy change to make.
remember the return value is defined after the -> so just add (greeting: String, language: String) after the ->.
be sure to name the member values of the tuple inside the return type. their data types will be strings.
then you need to return the actual tuple at the end of the function.
there are 2 constants, language and string, inside the function already. we will use those to make our tuple:
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
task 2 states: Create a variable named result and assign it the tuple returned from function greeting. (Note: pass the string "Tom" to the greeting function.)
easy enough. we need to create a new variable and assign it the return value of greeting, passing in "Tom" as the argument
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting("Tom")
finally task 3 states: Using the println statement, print out the value of the language element from the result tuple
you can access members of a tuple using their name and dot syntax
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting("Tom")
//used dot syntax to access the language member of result
println(result.language)

Anthony Walker
4,448 Pointsthanks for this. I thought I did this but must have had the syntax wrong. You have saved me throwing the computer out the window. This language is a little different from PHP and Javascript but getting there

Abdallah ElMenoufy
1,356 PointsHI,
I did the same as Anthony with task 2, here's my code but still stuck on error that the result variable has the wrong value, although I tried it on Xcode Playground and it worked just fine, Please advise guys:
func greeting(person: String) -> (greeting: String, language: String) { let language = "English" let greeting = "Hello (person)"
return (greeting, "Your language is: \(language)")
} var result = greeting("Tom")