Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial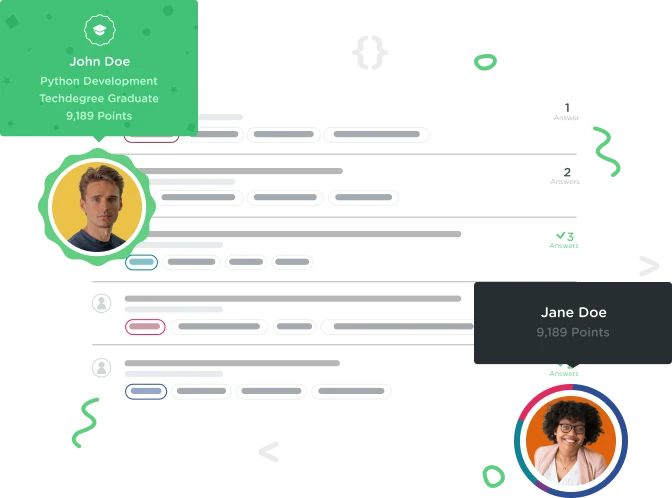

luckeday
6,123 PointsWhat is 'undefined' in my code?
Here is my code for the Build an Object Challenge, Part 2:
function print(message) {
document.write(message);
}
function profile(name, track, points, achv) {
print('Student: ' + name + '<br>');
print('<ol>Track: ' + track + '<br>');
print('Points: ' + points + '<br>');
print('Achievements: ' + achv + '</ol>');
}
var students = [
{ name: 'John', track: 'iOS', achievements: '30', points: '344' },
{ name: 'Shaun', track: 'Web Design', achievements: '20', points: '145'},
{ name: 'Martha', track: 'FED', achievements: '13', points: '453'},
{ name: 'Alice', track: 'Android', achievements: '35', points: '390' },
{ name: 'Stewart', track: 'CSS', achievements: '11', points: '470' }
];
for (var prop in students) {
print(profile
(students[prop].name,
students[prop].track,
students[prop].points,
students[prop].achievements)
);
}
Just before 'Student:' it comes up as undefined? What is causing this? I cannot find it for the life of me. Thank you.
2 Answers

Shawn Anderton
24,124 PointsYou don't need to use the print function in for loop. Your are already using it in the profile function. to print the data.
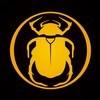
rydavim
18,814 PointsYou don't need to call the print function when you call profile, because profile already prints the information.
for (var prop in students) {
print(profile // Don't use print here, just call the profile function.
(students[prop].name,
students[prop].track,
students[prop].points,
students[prop].achievements)
);
}