Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial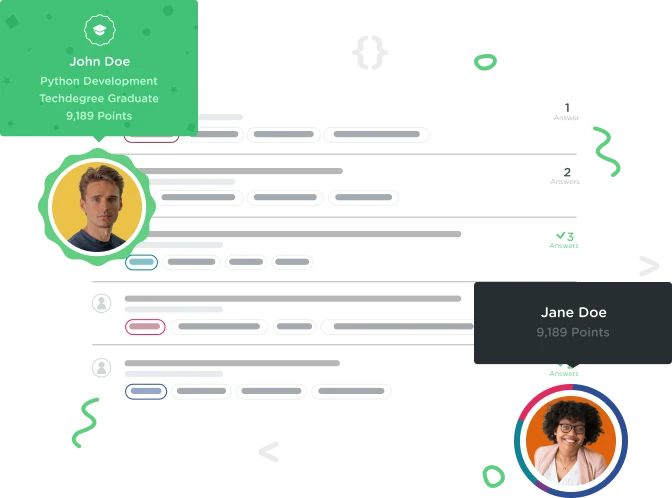

Tomasz Baranczuk
7,055 PointsWhat is wrong again?
I can not fing mistake in my code to complete the task.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int newLapsAmount = lapsDriven + laps;
barCount -= laps;
if (newLapsAmount > MAX_BARS) {
throw new IllegalArgumentException("Too many laps");
}
laps = newLapsAmount;
}
}
3 Answers
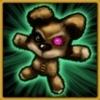
Dave Harker
Courses Plus Student 15,510 PointsHi Tomasz,
I approached it a little differently to Christian as I did not want laps updating if there was not enough power left. Only a slight difference but thought I'd post it here for you:
public void drive(int laps) {
if (barCount < laps) {
throw new IllegalArgumentException("Not enough power.");
} else {
lapsDriven += laps;
barCount -= laps;
}
}
Just an alternative. Hope it helps,
Dave.
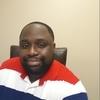
Christian Tisby
9,329 PointsHey Dave,
Could you explain what you mean when you say that you did not want laps updating if there was not enough power left? I had a time with getting this one right myself, so any additional explanation or insight would help me to get a clearer understanding. Thanks in advance!
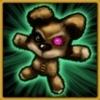
Dave Harker
Courses Plus Student 15,510 PointsHi Christian,
Could you explain what you mean when you say that you did not want laps updating if there was not enough power left?
On reflection I'm not entirely sure why I mentioned that as it's not applicable to the challenge scenario. I suppose because there are scenarios where the method code can run/complete after an exception is thrown (e.g. within a finally block) so why not make sure that if it shouldn't be updated, then it isn't be updated.
In saying that, feel free to ignore those ramblings and place the variable updates outside of the if statement. It will work just fine there also :)
Dave.

Tomasz Baranczuk
7,055 PointsWhy barCount, no MAX_BARS?
I start my GoKart sesion with full battery (8 bars). 1 lap = 1 bar.
When I count laps I count from 1, 2, 3, 4 ... 8 (last possible lap) Energy count going opposite 8, 7, 6, 5, ... 1
When battery has 3 bars I did already 5 laps (laps > barCount). It is looks for me like I stop my sesion now, so I can not use all bettery.
Please explein when I did mistake with my logic?
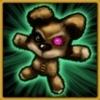
Dave Harker
Courses Plus Student 15,510 PointsHi Tomasz,
Because the laps being passed into the drive method are laps to do, not your lapsDriven (total laps completed).
So each lap you do (assuming you have the energy) increments your lapsDriven and decrements bars.
Consider this scenario. You start with a full battery (8 bars)
- Call drive method to drive 1 lap. laps(1) bars(8). OK increment lapsDriven by 1 (total 1). decrement bars by 1 (7remain)
- Call drive method to drive 3 lap. laps(3) bars(7). OK increment lapsDriven by 3 (total 4). decrement bars by 3 (4remain)
- Call drive method to drive 2 lap. laps(2) bars(4). OK increment lapsDriven by 2 (total 6). decrement bars by 2 (2remain)
- Call drive method to drive 3 lap. laps(3) bars(2). NOT OK. do nothing. no lapsDriven inc, no bars dec. try again
- Call drive method to drive 2 lap. laps(2) bars(2). OK increment lapsDriven by 2 (total 8). decrement bars by 2 (0remain)
If you want to do more laps you might need to call the chargeBattery method :) because as you can see, when you do 8 laps, you use 8 bars.
I hope helps clear it up. Happy coding,
Dave

Tomasz Baranczuk
7,055 PointsThis is beat more clear. Thank you.
Christian Tisby
9,329 PointsChristian Tisby
9,329 PointsI just got over this one myself.