Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial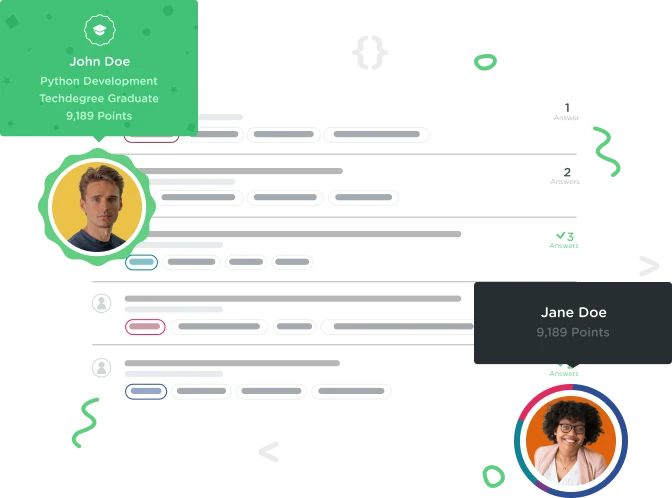

Amy Shah
25,337 PointsWhat is wrong here ?
I thought I was doing as the question asked.
using System.Web.Mvc;
using IssueReporter.Data;
using IssueReporter.Models;
namespace IssueReporter.Controllers
{
public class IssuesController : Controller
{
private IssuesRepository _issuesRepository;
public IssuesController()
{
_issuesRepository = new IssuesRepository();
}
public ActionResult Index()
{
return View();
}
public ActionResult Report()
{
var issue = new Issue();
SetupDepartmentsSelectListItems();
return View(issue);
}
[HttpPost]
public ActionResult Report(Issue issue)
{
if (issue.Severity == Issue.SeverityLevel.Critical && string.IsNullOrEmpty(issue.Email))
{
// TODO Use the ModelState.AddModelError method to add an error message for the "Email" field.
ModelState.AddModelError("Email", "The email is required for critical issues.");
}
if (ModelState.IsValid)
{
_issuesRepository.AddIssue(issue);
return RedirectToAction("Index");
}
SetupDepartmentsSelectListItems();
return View(issue);
}
private void SetupDepartmentsSelectListItems()
{
ViewBag.DepartmentsSelectListItems = new SelectList(
Data.Data.Departments, "Id", "Name");
}
}
}
1 Answer

James Churchill
Treehouse TeacherAmy,
You're really close! When I copied and pasted your solution into the code challenge, this was the error message that I received:
In the 'Report' POST action method, are you calling the 'ModelState.AddModelError' method with an 'errorMessage' parameter value of 'The Email field is required for critical issues.'?
You're definitely calling the ModelState.AddModelError
method, so that's not the problem. But are you passing the expected error message of "The Email field is required for critical issues."?
Thanks ~James