Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial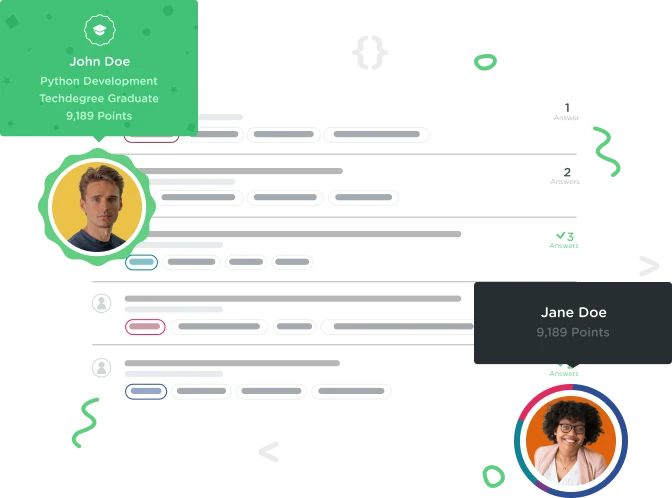

Navrozbek Akhmedov
1,106 PointsWhat is wrong here? Thanks in advance
There is compile error
public class MainActivity extends AppCompatActivity implements MainActivityView {
TextView counter;
Button button;
MainActivityPresenter presenter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
presenter = new MainActivityPresenter(this);
counter = (TextView) findViewById(R.id.textView);
button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int count = Integer.parseInt(counter.getText().toString());
presenter.updateTextViewText(Integer.toString(count + 1));
}
});
}
@Override
public void changeTextViewText(String text) {
counter.setText(text);
}
}
public interface MainActivityView {
void changeTextViewText(String text);
}
public class MainActivityPresenter {
MainActivityView view;
public void MainActivityPresenter(MainActivityView view) {
this.view = view;
}
public void updateTextViewText(String text) {
view.changeTextViewText(text);
}
}
1 Answer

Ben Deitch
Treehouse TeacherIn MainActivityPresenter you've added a return type to the constructor. Take that out and you've got it:
public class MainActivityPresenter {
MainActivityView view;
public MainActivityPresenter(MainActivityView view) {
this.view = view;
}
public void updateTextViewText(String text) {
view.changeTextViewText(text);
}
}
Hope that helps!