Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial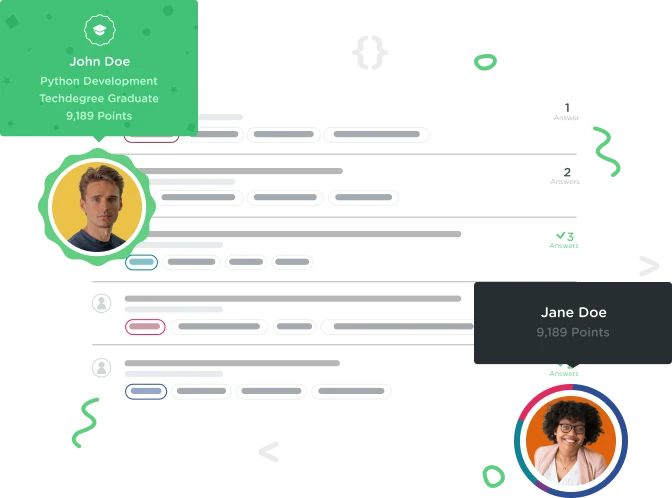

Aditya Gawade
1,182 Pointswhat is wrong? i did not understand the question. what is the procedure to create an instance?
Let's get in some practice creating a class. Declare a class named Shape with a variable property named numberOfSides of type Int.
Remember that with classes you are required to write an initializer method.
Once you have a class definition, create an instance and assign it to a constant named someShape.
// Enter your code below
class Shape () {
var numberOfSides : Int
let someShape = numberOfSides
}
4 Answers
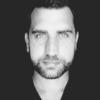
Jhoan Arango
14,575 PointsInitializing is the process of giving an object all it's properties an initial value. What do I mean by this ?
Let's say we want to create an object, in order for us to be able to create this object we need a "blue print", which sets the requirements for this object. This "blue print" we are going to call it a class.
Let's create a class that when fully initialized it will create a "Car", and this car will be the instance.
Example:
Here we have a class called AutoFactory, and this class has 3 properties that are optional they do not have any initial values other than "nil". As you can see the class has no initializer, so we can't give those properties any values at all so we won't be able to create an instance of this class.
class AutoFactory {
// Properties
let model: String?
let color: String?
let doors: Int?
}
let car = AutoFactory() // No initializer, so it won't create an instance.
Let's give this class an initializer:
class AutoFactory {
// Properties
let model: String?
let color: String?
let doors: Int?
// Initializer
init(model: String, color: String, doors: Int){
self.model = model
self.color = color
self.doors = doors
}
}
// Now we have an initializer and we can give those properties
// an initial value, and this will create an instance of the class.
let fordFiesta = AutoFactory(model: "Fiesta", color: "White", doors: 4)
// You can access these properties with the dot notation.
fordFiesta.color // Will print white
fordFiesta.doors // Will print 4
Hope this helps you.
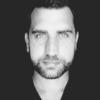
Jhoan Arango
14,575 PointsHello:
So, the challenge wants you to create a class. After creating the class, you will need to create an instance of it.
Let's start by creating a class and providing it with an initializer.
class Shape {
// Property
var numberOfSides: Int?
// Initializer
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
}
The initializer is what gives properties their values.
After you have created the class, then you can have an instance of it.
// Instance of Shape.
let square = Shape(numberOfSides: 4)
Good luck, if you don't understand please let me know.

Aditya Gawade
1,182 Pointsi am still having problems wrapping my head around the initializer concept. thank u for ur answer though
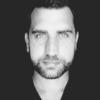
Jhoan Arango
14,575 PointsDo you need a better explanation still ? sorry I am getting back to you now.

Aditya Gawade
1,182 Pointsno problem at all. yes i do if you don't mind please. i do not understand the initialiser concept. i feel like its useless.
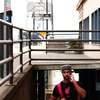
Devin Boddie
6,777 Points// This is the code that I used, but unfortunately it will not compile however I'm pretty sure that it is correct
class Shape {
var numberOfSides: Int
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
let someShape = Shape(numberOfSides: 3)
}
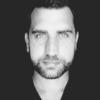
Jhoan Arango
14,575 PointsHello Davin:
What's happening with your code is that you are trying to create an instance of your Shape class within the class definition.
Try this:
class Shape { // This is the opening bracket of the class
var numberOfSides: Int
init(numberOfSides: Int) {
self.numberOfSides = numberOfSides
}
} // This is the closing bracket of the class
// This is outside of the class, now you should be able to create an instance.
let someShape = Shape(numberOfSides: 3)
Good luck
Gennaro Turco
2,557 PointsGennaro Turco
2,557 PointsThis is the kind of help we need to properly learn! Thank you very much!!
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsYou are very welcome. You can tag me if you need help in other questions.
Happy Coding