Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial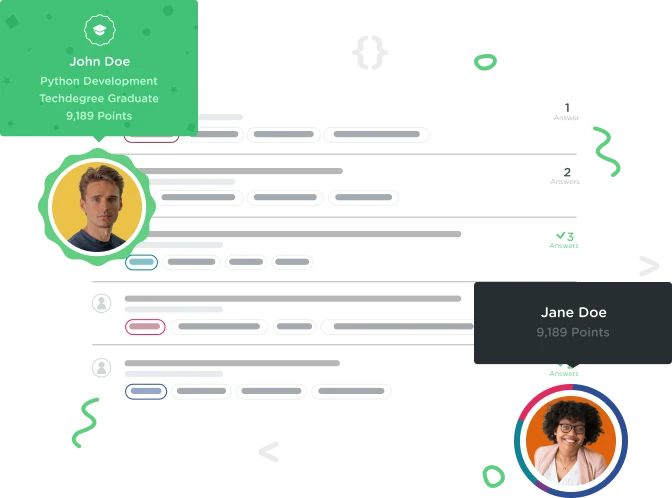
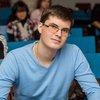
Ilia Galperin
6,522 PointsWhat is wrong in my code?
import random
import os
#draw grid
CELLS = [(0,0), (1,0), (2,0), (3,0), (4,0),
(0,1), (1,1), (2,1), (3,1), (4,1),
(0,2), (1,2), (2,2), (3,2), (4,2),
(0,3), (1,3), (2,3), (3,3), (4,3),
(0,4), (1,4), (2,4), (3,4), (4,4)]
def clear_screen():
os.system('cls' if os.name == 'nt' else 'clear')
def get_location():
return random.sample(CELLS, 3)
def move_player(player, move):
x, y = player
if move == 'LEFT':
x -= 1
if move == 'RIGHT':
x += 1
if move == 'UP':
y -= 1
if move == 'DOWN':
y += 1
return player
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
return x, y
monster, door, player = get_location()
while True:
valid_moves = get_moves(player)
clear_screen()
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player))
print("You can make the following moves {}".format(", ".join(valid_moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in valid_moves:
player = move_player(player, move)
else:
print("\n ** Walls are Hard! Don't run into them **\n")
continue
I have a mistake :
Welcome to the dungeon!
You're currently in room (0, 1)
Traceback (most recent call last):
File "dungeon_game.py", line 56, in <module>
print("You can make the following moves {}".format(", ".join(valid_moves)))
TypeError: sequence item 0: expected str instance, int found
2 Answers

Ullas Savkoor
6,028 PointsHi, The error in your code is in the function get_moves(player), the function should return the list 'moves' instead you are returning a tuple of integers x,y. Thus the variable instead of containing list of moves instead contains a tuple of integers.
@Charles Kenney: python dynamically determines the type of variables, you cannot define an integer statically. The reason the error is coming is because valid_moves contains tuple of integer. Casting the variable to string won't work.
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
return move
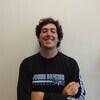
Charles Kenney
15,604 PointsYou got a type error because you used an integer 'valid_moves' in your string interpolation on line 56! You can simply force valid_moves as a string by doing the following...
print("You can make the following moves {}".format(", ".join(str(valid_moves))))
your program will then output:
Welcome to the dungeon!
You're currently in room (3, 1)
You can make the following moves (, 3, ,, , 1, )
Enter QUIT to quit
Cheers, Charles