Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial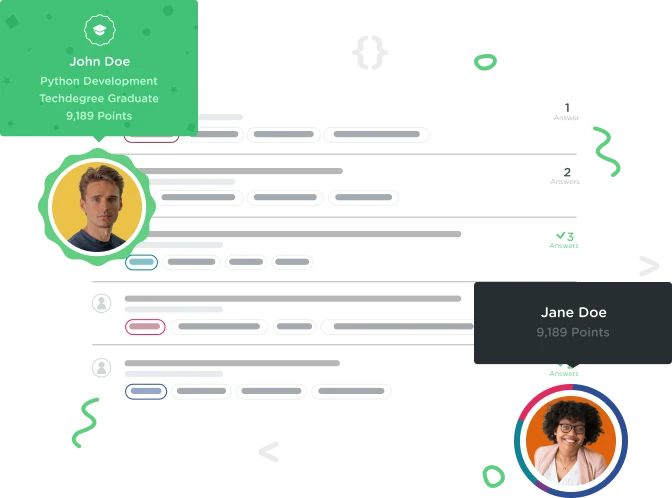
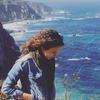
Deniz Kahriman
8,538 PointsWhat is wrong in this code? Trying to count the words in a string and assign values. Thank you!
Trying to complete the challenge but got stuck. Thank you very much in advance!
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
final_list = {}
for word in string.split():
if word.lowercase() in string.lowercase():
final_list[word] += 1
else:
final_list[word] =1
return final_list
2 Answers
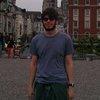
Robert Stefanic
35,170 PointsThere's an extra indentation in your if/else statement. The else
block is an extra level in, so it doesn't have an if
statement to match it.
Just bring your else
statement out so it lines up with your if
statement.
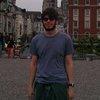
Robert Stefanic
35,170 PointsYou're welcome.
Deniz Kahriman
8,538 PointsDeniz Kahriman
8,538 PointsThanks, Robert! I tried it but still didn't work :(
Robert Stefanic
35,170 PointsRobert Stefanic
35,170 PointsI see. There are a few things to note here:
First, lowercase() isn't a method strings have. The method you're looking for is
lower()
.So change and
.lowercase()
to.lower()
.Second, in your condition for your
if
statement, you're checking the wrong thing:You're checking to see if the lower case word is in the string that you pulled the word from! You need to check to see if the word is part of your
final_list
dictionary.And lastly, when you add the words to your dictionary, you should lowercase them. The example has the word 'sam' in the dictionary as a lower case word, even though it appears capitalized in the string that's passed to the function.
So just make sure that you add
.lower()
to the keywords that you're adding to your dictionary, like so:final_list[word.lower()] += 1
Deniz Kahriman
8,538 PointsDeniz Kahriman
8,538 PointsHi Robert,
I tried it again based on your notes below but I'm still getting an error. Would you mind looking at the code below to see what I'm missing here? Thanks!
Robert Stefanic
35,170 PointsRobert Stefanic
35,170 PointsYou're very close. Think about this: you're calling
.lower()
onfinal_list
. How could you apply.lower()
to a dictionary object? You can't.You've already lower cased the words before adding them to your final_list. Since they're already lowered, there's no need to lowercase the words in final_list because they're already entered into your dictionary as lower cased words!
So just remove
.lower()
from final list. :)Deniz Kahriman
8,538 PointsDeniz Kahriman
8,538 PointsFinally, completed the challenge! Thank you very much!! :)