Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial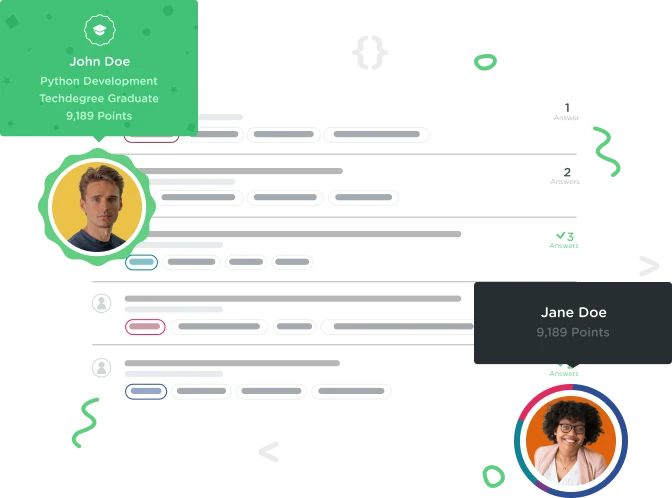
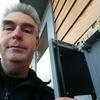
Remco de Wilde
9,864 PointsWhat is Wrong in this unit test?
I get this error Did you declare a method named MapTest in MapTests
But compiler does not give any error :S
namespace TreehouseDefense
{
public class Map
{
public readonly int Width;
public readonly int Height;
public Map(int width, int height)
{
if(width < 1 || height < 1)
{
throw new System.ArgumentOutOfRangeException(
"Map must be at least 1x1");
}
Width = width;
Height = height;
}
public bool OnMap(Point point)
{
return point.X >= 0 && point.X < Width &&
point.Y >= 0 && point.Y < Height;
}
}
}
using Xunit;
namespace TreehouseDefense.Tests
{
public class MapTests
{
[Fact]
public void OnMapTest()
{
var target = new Map(3, 3);
var point = new Point(0, 0);
Assert.True(target.OnMap(point));
}
}
public class MapTest
{
[Fact]
public void OutOfBoundsExceptionTest()
{
var map = new Map(3, 3);
Assert.Throws<OutOfBoundsException>(()=>new MapLocation (3,3, map));
}
}
}
using System;
namespace TreehouseDefense
{
public class Point
{
public readonly int X;
public readonly int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
public double DistanceTo(Point point)
{
return Math.Sqrt(Math.Pow(X - point.X, 2.0) + Math.Pow(Y - point.Y, 2.0));
}
}
}
2 Answers
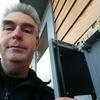
Remco de Wilde
9,864 Pointsthinking way to far (looking at the video)
Only line of code i need was
{
Assert.Throws<ArgumentOutOfRangeException>(() =>new Map(0, 0));
}
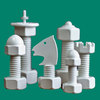
Steven Parker
231,545 PointsYour code may compile, but you did something other than what the task asked.
The challenge task is to "Add a test method named MapTest", but it looks like you added a method named OutOfBoundsExceptionTest, in a new class named MapTest. The challenge probably expects the new method to be added to the existing class MapTests (plural).
The task also says your test should throw "the ArgumentOutOfRangeException when the width or height is too small.", but you test seems to throw the OutOfBoundsException instead.
Did you paste in something from another source to use as a model and then forget to modify it to conform to the challenge?
Steven Parker
231,545 PointsSteven Parker
231,545 PointsCongratulations, and good job!
I hope my answer was helpful.