Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial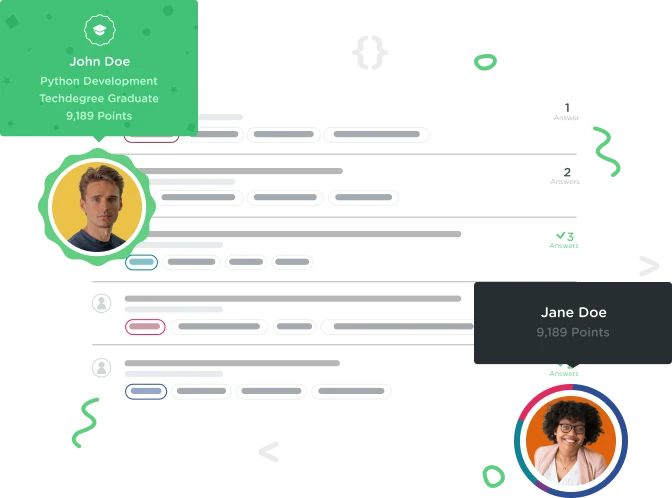
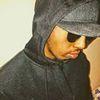
ibrahim Warsame
7,803 PointsWhat is wrong? please help.
I adde Appels, crim, eggs to my list, and when it asked me where to put it i pressed enter, but when i typed SHOW it didnt show my list. Whay is that?.
here is my code:
shopping_list = []
def show_help():
print("\nSeprate each item with a comma.")
print("Type DONE to quit, SHOW to see the current list, and HELP to see this massage")
def show_list():
count = 1
for item in shopping_list:
"{}: {}".format(count, item)
count += 1
print("Give me a list of things you want to shop for")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
print(shopping_list)
continue
elif new_stuff == "DONE":
print(shopping_list)
break
else:
new_list = new_stuff.split(",")
index = input("Add this at a certin spot? press enter for the end of the list, "
"or give me a number. Currently {} items in the list".format(len(shopping_list)))
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
3 Answers
Aaron Kaye
10,948 PointsPython can be a PAIN at times, I finally figured it out though. You need to un-indent the final else, right now it is indented with the for loop so since you aren't specifying an index and the else is indented into the if, it is never actually adding to the list.
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
Other notes, Your show_list() method needs a print:
print("{}: {}".format(count, item)) #Added Print
You need to specify show_list() when SHOW command is given, right now you say print:
elif new_stuff == "SHOW":
show_list()# changed
continue
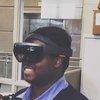
Guled Ahmed
12,806 PointsEdit: Aaron Kayes answer is perfect!
Hey Ibrahim! How are you? I found the problem with your 'SHOW' command. I tested your code and I found that you accidently placed an extra tab in your If statement.
Original
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
Fixed Version
if index:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else:
for item in new_list:
shopping_list.append(item.strip())
In the fixed version, I took away some spaces from the else statement so that the if and else keywords are aligned. Python is very tricky at times when it comes to indentation, I make this mistake a lot. You'll get use to it with practice. Keep up the great work!
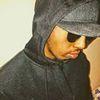
ibrahim Warsame
7,803 PointsI really appreciate helping me out. Thanks for the help ,.
Aaron Kaye
10,948 PointsAaron Kaye
10,948 PointsAlso, a general debugging tip is it is useful to throw print statements in to see if your code makes it to that section. For example, to debug this I knew we didn't specify an index so it would need to be the else case. I then added a print statement in the else that would print "I RAN" to the console. I reran the code and notice that was never printing (meaning my code would never get to that point like I expected), so I tried work my way up from there. Just general debugging tip which you may have even already tried! Good luck studying Python!