Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial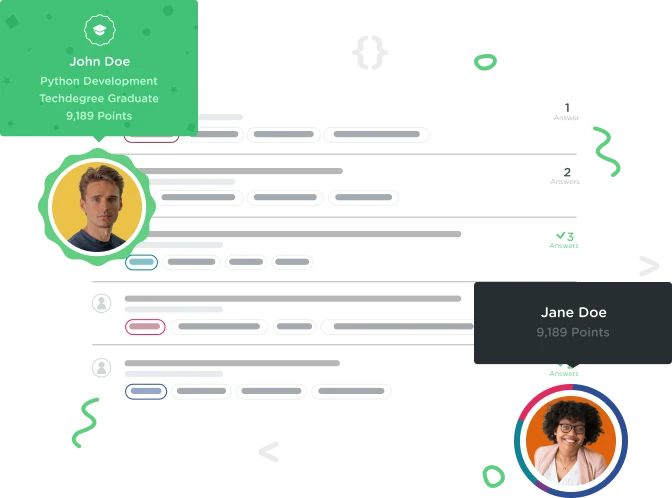

Jesse Richards
13,964 PointsWhat is Wrong so Fustrated???
Error???
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
CourseStudent Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<Student> Students { get; set; }
}
}
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class CourseStudent
{
public int Id { get; set; }
public int CourseId { get; set; }
public int StudentId { get; set; }
public decimal Grade { get; set; }
public Course Course { get; set; }
public Student Student { get; set; }
}
}
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Student
{
public Student()
{
Courses = new List<Course>();
}
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Course> Courses { get; set; }
}
}

Jesse Richards
13,964 PointsCourse.cs(9,38): error CS0029: Cannot implicitly convert type System.Collections.Generic.List' to
Treehouse.CodeChallenges.CourseStudent'
Compilation failed: 1 error(s), 0 warnings
Challenge Task 2 of 3
Now that we have an explicit bridge entity class, let's update the Course and Student classes to make use of it. In Course.cs make the following changes to the Course class: Update the Students navigation collection property to be of type CourseStudent Update the default constructor to initialize the Students property to an instance of List<CourseStudent

Harrison Brock
1,363 PointsLooks like your problem is here:
CourseStudent Students = new List<CourseStudent>();
Should be:
List<CourseStudent Students = new List<CourseStudent>();
3 Answers
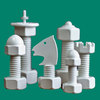
Steven Parker
231,275 PointsI see two issues:
I'm assuming you're on task 2. Now on one line you have this:
CourseStudent Students = new List<CourseStudent>();
You've updated the instance correctly, but you are just assigning, not declaring here. So Students doesn't need a type in front of it.
public ICollection<Student> Students { get; set; }
The instructions said, "Update the Students
navigation collection property to be of type CourseStudent
", but your collection is still of type "Student" here.
So basically, if you start with the original contents of Course.cs, you just replace the word "Student" (singular) with "CourseStudent" in two places for task 2. Task 3 will be very similar but you'll replace a different word in another file.

Jesse Richards
13,964 PointsHarrison when I did what you said i got this " Bummer! Is Course.Students of type System.Collections.Generic.ICollection<Treehouse.CodeChallenges.CourseStudent>?"
and Steven i played with what you said and i dont really know how to arrange it, it isn't working imma go away from it for a little getting very fustrated and watch the video 3X now and can't find what im missing?!?
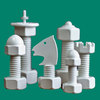
Steven Parker
231,275 PointsIt goes to 3X? I've never gotten it past 2X! But I added another hint to my answer above.

Jesse Richards
13,964 Pointso my word... thank you so much Steven so I can't believe sometimes how difficult i make this to end up being easy, i guess thats why programmers make the big bucks lolz thank again!!!
Harrison Brock
1,363 PointsHarrison Brock
1,363 PointsWhat is your errors?